webRTC vue案例
时间: 2023-11-20 11:07:25 浏览: 103
作为AI助手,我需要先声明一下:WebRTC技术是一种实时通信的技术,用于浏览器之间的音视频通话、屏幕共享等场景。而Vue是一种前端框架,用于构建用户界面。
因此,WebRTC和Vue并不是一种直接相关的技术,但是可以通过Vue来构建WebRTC应用的用户界面。
下面是一个简单的WebRTC Vue案例:
1. 安装Vue和WebRTC相关的库
```
npm install vue webrtc-adapter vue-webrtc
```
2. 在Vue组件中引入WebRTC组件
```html
<template>
<div>
<video ref="localVideo" autoplay muted></video>
<video ref="remoteVideo" autoplay></video>
<button @click="startCall">Start Call</button>
<button @click="endCall">End Call</button>
</div>
</template>
<script>
import VueWebRTC from 'vue-webrtc'
export default {
name: 'WebRTCComponent',
components: {
VueWebRTC
},
data () {
return {
localStream: null,
remoteStream: null,
isCalling: false
}
},
methods: {
async startCall () {
this.isCalling = true
this.localStream = await navigator.mediaDevices.getUserMedia({ audio: true, video: true })
this.$refs.localVideo.srcObject = this.localStream
const rtcPeerConnection = new RTCPeerConnection({
iceServers: [
{ urls: 'stun:stun.stunprotocol.org' }
]
})
this.localStream.getTracks().forEach(track => rtcPeerConnection.addTrack(track, this.localStream))
rtcPeerConnection.addEventListener('icecandidate', event => {
if (event.candidate) {
// send ice candidate to remote peer
}
})
rtcPeerConnection.addEventListener('track', event => {
this.remoteStream = event.streams[0]
this.$refs.remoteVideo.srcObject = this.remoteStream
})
// create offer and set local description
const offer = await rtcPeerConnection.createOffer()
await rtcPeerConnection.setLocalDescription(offer)
// send offer to remote peer
},
endCall () {
// close peer connection and release stream resources
this.isCalling = false
this.localStream.getTracks().forEach(track => track.stop())
this.remoteStream.getTracks().forEach(track => track.stop())
const rtcPeerConnection = this.$refs.vueWebRTC.rtcPeerConnection
if (rtcPeerConnection) {
rtcPeerConnection.close()
}
}
}
}
</script>
```
3. 在Vue实例中使用WebRTC组件
```js
import Vue from 'vue'
import WebRTCComponent from './WebRTCComponent.vue'
new Vue({
el: '#app',
components: { WebRTCComponent },
template: '<WebRTCComponent/>'
})
```
在这个案例中,我们使用了vue-webrtc组件来简化WebRTC的使用。具体来说,我们在startCall方法中使用getUserMedia获取本地音视频流,创建RTCPeerConnection实例,并将本地流添加到peer connection中。然后,我们使用createOffer方法创建一个offer并将其设置为本地的SDP(Session Description Protocol)。最后,我们将offer发送给远程peer,并等待远程peer的answer。在answer到达之后,我们将其设置为远程SDP,并完成peer connection的建立。
需要注意的是,在实际应用中,我们需要处理各种事件,例如网络中断、peer connection失败等等。同时,我们还需要考虑如何安全地传输音视频流以及如何支持多人通话等场景。
阅读全文
相关推荐
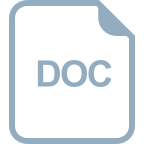
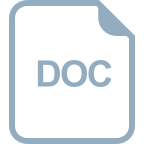
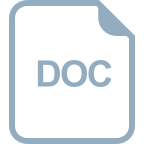

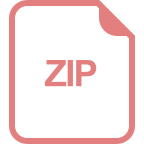
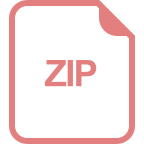
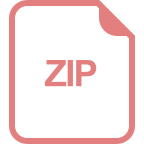
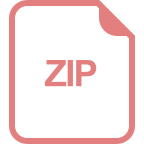
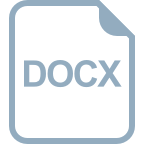
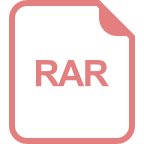
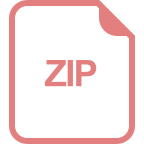
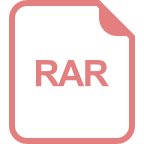
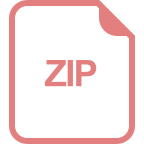
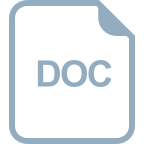
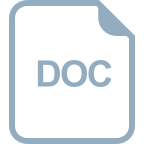

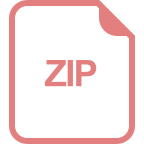
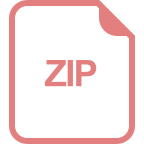
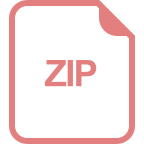