在链式存储结构上,将x插入到单链表的第i个数据元素位置上(用C语言)
时间: 2023-05-26 21:07:37 浏览: 98
以下是将x插入到单链表的第i个数据元素位置的C语言代码实现:
```c
// 定义链表节点数据类型
struct ListNode {
int val;
struct ListNode *next;
};
// 将元素 x 插入单链表的第 i 个位置上
struct ListNode* insert(struct ListNode* head, int i, int x) {
// 新建节点
struct ListNode* newNode = (struct ListNode*)malloc(sizeof(struct ListNode));
newNode->val = x;
newNode->next = NULL;
if (head == NULL || i <= 0) {
// 链表为空或插入位置为0,则插入到头节点位置
newNode->next = head;
head = newNode;
} else {
// 找到插入位置的前一个节点
struct ListNode* pre = head;
int pos = 1;
while (pre != NULL && pos < i - 1) {
pre = pre->next;
pos++;
}
if (pre == NULL) {
// i 超出链表长度,插入到链表尾部
pre = head;
while (pre->next != NULL) {
pre = pre->next;
}
pre->next = newNode;
} else {
// 插入到中间位置
newNode->next = pre->next;
pre->next = newNode;
}
}
return head;
}
```
使用示例:
```c
int main() {
// 创建链表 1->2->4->5
struct ListNode* head = (struct ListNode*)malloc(sizeof(struct ListNode));
head->val = 1;
head->next = (struct ListNode*)malloc(sizeof(struct ListNode));
head->next->val = 2;
head->next->next = (struct ListNode*)malloc(sizeof(struct ListNode));
head->next->next->val = 4;
head->next->next->next = (struct ListNode*)malloc(sizeof(struct ListNode));
head->next->next->next->val = 5;
head->next->next->next->next = NULL;
// 插入元素3到第3个位置
head = insert(head, 3, 3);
// 遍历链表,输出结果 1->2->3->4->5
struct ListNode* p = head;
while (p != NULL) {
printf("%d ", p->val);
p = p->next;
}
// 释放链表空间
while (head != NULL) {
struct ListNode* tmp = head;
head = head->next;
free(tmp);
}
return 0;
}
```
阅读全文
相关推荐



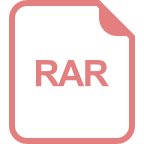







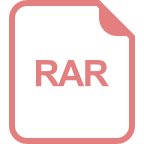


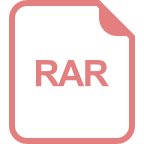



