创建XML文件并压缩,不保存到本地,生成字节流
时间: 2024-01-08 17:02:11 浏览: 113
可以使用Java中的DOM API来创建XML文件,并使用Java中的压缩流API将其压缩成字节流,最终将其发送给用户。以下是一个示例代码:
```java
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// 创建XML文档
DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
DocumentBuilder builder = factory.newDocumentBuilder();
Document doc = builder.newDocument();
// 创建XML元素
Element root = doc.createElement("root");
doc.appendChild(root);
Element child1 = doc.createElement("child1");
child1.setTextContent("This is child1");
root.appendChild(child1);
Element child2 = doc.createElement("child2");
child2.setTextContent("This is child2");
root.appendChild(child2);
// 将XML文档转换成字节数组
TransformerFactory transformerFactory = TransformerFactory.newInstance();
Transformer transformer = transformerFactory.newTransformer();
ByteArrayOutputStream bos = new ByteArrayOutputStream();
transformer.transform(new DOMSource(doc), new StreamResult(bos));
byte[] xmlBytes = bos.toByteArray();
// 压缩XML字节数组
ByteArrayOutputStream compressedBos = new ByteArrayOutputStream();
ZipOutputStream zos = new ZipOutputStream(compressedBos);
zos.putNextEntry(new ZipEntry("file.xml"));
zos.write(xmlBytes);
zos.closeEntry();
zos.close();
byte[] compressedBytes = compressedBos.toByteArray();
// 设置响应头信息
response.setContentType("application/zip");
response.setHeader("Content-Disposition", "attachment;filename=myfile.zip");
// 将压缩后的字节数组写入响应输出流中
OutputStream out = response.getOutputStream();
out.write(compressedBytes);
out.flush();
out.close();
}
```
在上面的代码中,我们首先使用DOM API创建了一个XML文档。然后,我们将XML文档转换成字节数组,并使用ZipOutputStream将其压缩成字节数组。最后,我们设置响应头信息,指定要下载的文件名,并将压缩后的字节数组写入响应输出流中,完成文件的发送。
需要注意的是,以上代码中创建的XML文件比较简单,如果需要创建更复杂的XML文件,可以使用Java中的DOM API或者其他XML API来实现。
阅读全文
相关推荐
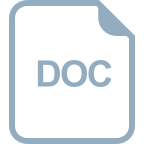
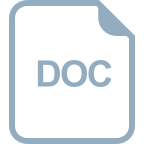
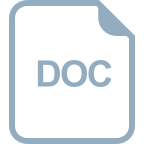

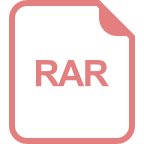
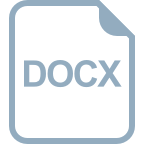
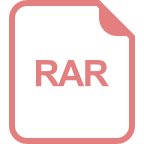
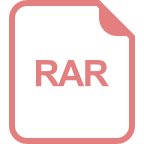
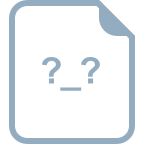
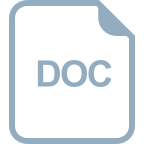
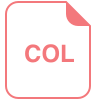
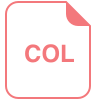
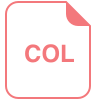
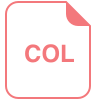
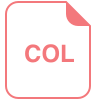
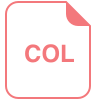
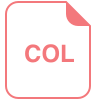
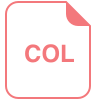
