keras如何调整模型使用GPU训练
时间: 2023-09-17 19:08:43 浏览: 116
在 Keras 中,可以通过设置环境变量 `CUDA_VISIBLE_DEVICES` 来指定使用的 GPU 设备编号。例如,如果有两个 GPU 设备,可以通过以下方式指定使用第二个 GPU 设备进行训练:
```
import os
os.environ["CUDA_VISIBLE_DEVICES"] = "1"
```
此外,还需要在 Keras 中设置使用 GPU 进行训练,可以通过以下代码实现:
```
import tensorflow as tf
from keras.backend.tensorflow_backend import set_session
config = tf.ConfigProto()
config.gpu_options.allow_growth = True # 允许 GPU 按需分配显存
set_session(tf.Session(config=config))
```
这样就可以在 Keras 中使用 GPU 进行模型训练了。需要注意的是,如果使用 TensorFlow 2.x 版本,可以直接使用 `tf.config.experimental.set_visible_devices` 来设置使用的 GPU 设备,而不需要设置环境变量 `CUDA_VISIBLE_DEVICES`。
相关问题
keras使用gpu训练模型
Keras是一个高级神经网络API,它可以在TensorFlow、Theano和CNTK等后端运行。如果你想利用GPU进行模型训练,首先你需要确保你的系统上安装了支持GPU的版本的Python(如anaconda)和相应的深度学习库。
以下是使用Keras在GPU上训练模型的基本步骤:
1. **安装必要的库**:
- 安装`tensorflow-gpu`或`keras`(如果已经包含了TensorFlow):```pip install tensorflow-gpu```
或者直接安装`keras`:```pip install keras```
2. **检查硬件**:
在终端或命令提示符下输入 `import tensorflow as tf; print(tf.test.gpu_device_name())`,确认GPU是否可用。
3. **设置会话**:
```python
import tensorflow as tf
config = tf.ConfigProto()
config.gpu_options.allow_growth = True # 动态分配GPU内存
sess = tf.Session(config=config)
K.set_session(sess) # 将会话绑定给Keras
```
4. **创建模型**:
使用Keras API定义你的模型,比如一个简单的卷积神经网络(CNN)或者全连接层(Dense)网络。
5. **模型编译**:
设定损失函数、优化器和评估指标,并编译模型。
6. **数据预处理**:
准备好你的训练数据,可以使用Keras提供的ImageDataGenerator等工具进行数据增强。
7. **模型训练**:
调用 `.fit()` 方法,传入训练集、验证集以及一些训练参数,模型会在GPU上进行训练。
```python
model.fit(x_train, y_train,
batch_size=32,
epochs=10,
validation_data=(x_val, y_val))
```
keras如何使用GPU训练
要使用GPU训练Keras模型,您需要确保您的计算机具有NVIDIA GPU,并且您已经安装了CUDA和cuDNN库。接下来,您可以按照以下步骤在Keras中使用GPU训练模型:
1. 导入所需的库和模块:
```
import tensorflow as tf
from tensorflow import keras
```
2. 设置Keras的后端为TensorFlow并配置GPU:
```
config = tf.compat.v1.ConfigProto()
config.gpu_options.allow_growth = True
session = tf.compat.v1.Session(config=config)
keras.backend.set_session(session)
```
3. 构建和编译您的Keras模型:
```
model = keras.Sequential()
# add layers to your model
model.compile(loss='binary_crossentropy', optimizer='adam', metrics=['accuracy'])
```
4. 使用GPU训练模型:
```
model.fit(x_train, y_train, epochs=10, batch_size=32)
```
请注意,在使用GPU训练模型时,您应该使用较大的批量大小和更多的训练时期,以充分利用GPU的性能。
阅读全文
相关推荐
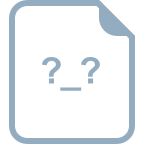
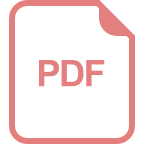
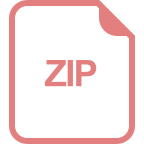
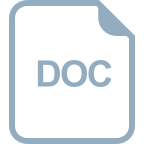











