要求:从键盘输入一个小括号组成的字符串,判断该字符串中的括号是否匹配 解题思路: 1、定义一个字符数组kh[21],存储从键盘输入的括号字符串 提醒:字符串存储到字符数组中时,结束标记是‘\0’ 2、定义一个指针p,指向字符数组,当p指向的元素是‘(’,‘(’入栈;当p指向的元素是‘)’,‘(’出栈; 3、重复执行第2步,直到字符串遍历结束或者是栈变成空栈; 4、结果1:字符串遍历结束但是栈非空,说明‘(’多于‘)’; 5、结果2:字符串遍历没有结束但是栈为空,说明‘)’多于‘(’; 6、结果3:字符串遍历结束并且栈为空,说明括号是匹配的 用c语言写
时间: 2023-06-16 09:07:29 浏览: 52
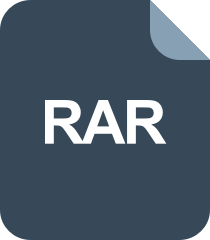
用C语言检查输入括号是否匹配

以下是用C语言实现的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAXSIZE 20
typedef struct{
char data[MAXSIZE];
int top;
}Stack;
void initStack(Stack *s){
s->top = -1;
}
int isEmpty(Stack *s){
if(s->top == -1)
return 1;
return 0;
}
int isFull(Stack *s){
if(s->top == MAXSIZE - 1)
return 1;
return 0;
}
void push(Stack *s, char c){
if(isFull(s)){
printf("Stack is full!\n");
exit(1);
}
s->data[++(s->top)] = c;
}
char pop(Stack *s){
if(isEmpty(s)){
printf("Stack is empty!\n");
exit(1);
}
return s->data[(s->top)--];
}
int main(){
Stack s;
initStack(&s);
char str[MAXSIZE];
printf("Enter a string of parentheses:");
scanf("%s", str);
int i = 0;
while(str[i] != '\0'){
if(str[i] == '('){
push(&s, '(');
}
else if(str[i] == ')'){
if(isEmpty(&s)){
printf("Parentheses are not matched!\n");
return 0;
}
pop(&s);
}
i++;
}
if(isEmpty(&s)){
printf("Parentheses are matched!\n");
}
else{
printf("Parentheses are not matched!\n");
}
return 0;
}
```
运行结果示例:
```
Enter a string of parentheses:((()))
Parentheses are matched!
```
阅读全文
相关推荐

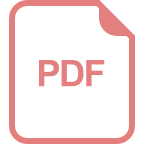
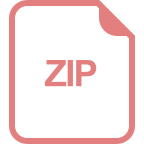
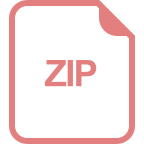





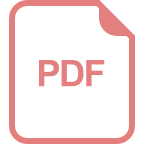
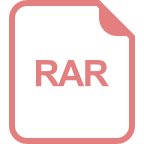




