要求:从键盘输入一个小括号组成的字符串,判断该字符串中的括号是否匹配 解题思路: 1、定义一个字符数组kh[21],存储从键盘输入的括号字符串 提醒:字符串存储到字符数组中时,结束标记是‘\0’ 2、定义一个指针p,指向字符数组,当p指向的元素是‘(’,‘(’入栈;当p指向的元素是‘)’,‘(’出栈; 3、重复执行第2步,直到字符串遍历结束或者是栈变成空栈; 4、结果1:字符串遍历结束但是栈非空,说明‘(’多于‘)’; 5、结果2:字符串遍历没有结束但是栈为空,说明‘)’多于‘(’; 6、结果3:字符串遍历结束并且栈为空,说明括号是匹配的
时间: 2023-06-16 08:07:27 浏览: 81
以下是一个示例代码,实现了输入小括号组成的字符串并判断括号是否匹配:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义栈结构体
typedef struct {
char data[21]; // 存储括号的字符数组
int top; // 栈顶指针
} Stack;
// 初始化栈
void init(Stack *s) {
s->top = -1;
}
// 入栈
void push(Stack *s, char c) {
if (s->top == 20) {
printf("Stack is full!\n");
exit(1);
}
s->data[++(s->top)] = c;
}
// 出栈
char pop(Stack *s) {
if (s->top == -1) {
printf("Stack is empty!\n");
exit(1);
}
return s->data[(s->top)--];
}
int main() {
char kh[21]; // 存储括号的字符数组
Stack s; // 定义栈
char *p = kh; // 指针p指向字符数组kh
int i = 0; // 计数器
printf("Please input the string of parentheses (no more than 20):\n");
scanf("%s", kh); // 输入括号字符串
init(&s); // 初始化栈
while (*p != '\0') { // 遍历字符数组
if (*p == '(') {
push(&s, *p); // 左括号入栈
} else if (*p == ')') {
if (s.top == -1) {
printf("Parentheses are not matched!\n");
exit(1);
} else {
pop(&s); // 右括号出栈
}
}
p++; // 指针p移动到下一个字符
i++; // 计数器加1
}
if (s.top == -1 && i <= 20) {
printf("Parentheses are matched!\n");
} else if (s.top != -1) {
printf("Parentheses are not matched!\n");
} else {
printf("Input string is too long!\n");
}
return 0;
}
```
在代码中,我们使用了一个栈结构体来存储左括号,当遍历到右括号时,如果栈非空,则出栈一个左括号,否则说明括号不匹配。最终,根据栈是否为空和输入字符串的长度来判断括号是否匹配。
相关推荐
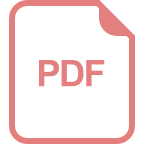
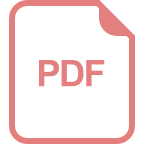
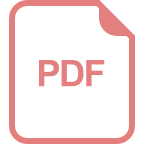














