c++ powerpoint 插件 文本框中插入公式 实例
时间: 2024-03-10 16:45:16 浏览: 168
以下是使用 C++ 在 PowerPoint 文本框中插入公式的示例代码:
```c++
#include <windows.h>
#include <atlbase.h>
#include <atlcom.h>
#include <atlstr.h>
#include <comutil.h>
#include <msppt.h>
#pragma comment(lib, "ole32.lib")
#pragma comment(lib, "oleaut32.lib")
int main()
{
// 初始化 COM 库
HRESULT hr = CoInitialize(NULL);
if (FAILED(hr))
{
return 1;
}
// 创建 PowerPoint 应用程序对象
CComPtr<_Application> pApp;
hr = pApp.CoCreateInstance(__uuidof(Application));
if (FAILED(hr))
{
CoUninitialize();
return 1;
}
// 创建演示文稿对象
CComPtr<_Presentation> pPresentation;
hr = pApp->Presentations->Add(msoTrue, &pPresentation);
if (FAILED(hr))
{
pApp->Quit();
CoUninitialize();
return 1;
}
// 创建幻灯片对象
CComPtr<Slide> pSlide;
hr = pPresentation->Slides->Add(1, ppLayoutText, &pSlide);
if (FAILED(hr))
{
pPresentation->Close();
pApp->Quit();
CoUninitialize();
return 1;
}
// 创建文本框对象
CComPtr<Shape> pShape;
hr = pSlide->Shapes->AddTextbox(msoTextOrientationHorizontal, 100, 100, 400, 200, &pShape);
if (FAILED(hr))
{
pPresentation->Close();
pApp->Quit();
CoUninitialize();
return 1;
}
// 插入公式
CComPtr<TextFrame> pTextFrame;
hr = pShape->TextFrame->QueryInterface(&pTextFrame);
if (FAILED(hr))
{
pPresentation->Close();
pApp->Quit();
CoUninitialize();
return 1;
}
CString strFormula = _T("x = \\frac{-b \\pm \\sqrt{b^2-4ac}}{2a}");
CComBSTR bstrFormula = strFormula;
CComPtr<Font> pFont;
hr = pTextFrame->TextRange->InsertAfter(bstrFormula, &pFont);
if (FAILED(hr))
{
pPresentation->Close();
pApp->Quit();
CoUninitialize();
return 1;
}
// 保存演示文稿
hr = pPresentation->SaveAs(CComBSTR(_T("test.pptx")), ppSaveAsOpenXMLPresentation, msoTrue);
if (FAILED(hr))
{
pPresentation->Close();
pApp->Quit();
CoUninitialize();
return 1;
}
// 退出 PowerPoint 应用程序
pPresentation->Close();
pApp->Quit();
CoUninitialize();
return 0;
}
```
在代码中,我们使用 PowerPoint 的 COM 接口来创建 PowerPoint 应用程序对象、演示文稿对象、幻灯片对象和文本框对象,然后通过 TextRange 对象的 InsertAfter 方法来插入公式。需要注意的是,公式使用 LaTeX 格式,需要在字符串前面加上 `_T` 宏来转换为 Unicode 字符串。另外,需要在项目属性中的 C/C++ -> 预处理器 -> 预处理器定义中添加 `_ATL_CSTRING_EXPLICIT_CONSTRUCTORS` 宏来支持 CString 类型。
阅读全文
相关推荐
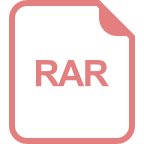
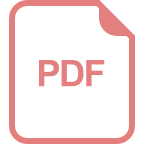





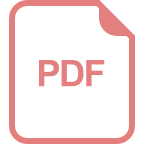
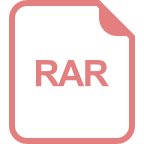
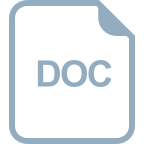
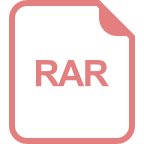
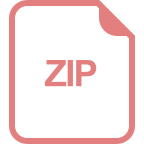
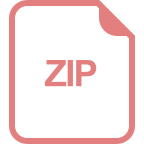
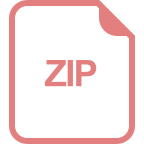
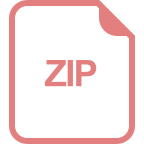