提供一个简单的登录页面的示例代码,前端使用HTML、CSS和JavaScript,后端使用Node.js和Express框架,读取本地Excel数据使用了xlsx库
时间: 2024-03-24 20:38:02 浏览: 73
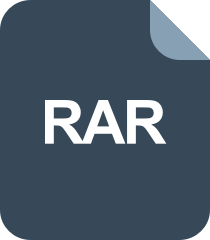
一个简单的html登录页面
好的,以下是一个简单的登录页面示例代码:
```html
<!DOCTYPE html>
<html>
<head>
<title>Login Page</title>
<link rel="stylesheet" type="text/css" href="style.css">
</head>
<body>
<div class="login-box">
<h2>Login</h2>
<form>
<label>Username</label>
<input type="text" name="username" id="username" required>
<label>Password</label>
<input type="password" name="password" id="password" required>
<button type="button" onclick="login()">Login</button>
</form>
</div>
<script src="script.js"></script>
</body>
</html>
```
接下来是样式表 `style.css`:
```css
.login-box {
width: 300px;
margin: auto;
margin-top: 100px;
padding: 20px;
border: 1px solid #ccc;
border-radius: 5px;
text-align: center;
}
h2 {
margin-top: 0;
}
label {
display: block;
margin-bottom: 5px;
text-align: left;
}
input[type="text"], input[type="password"] {
width: 100%;
padding: 5px;
margin-bottom: 10px;
border: 1px solid #ccc;
border-radius: 3px;
}
button {
background-color: #4CAF50;
color: #fff;
padding: 5px 10px;
border: none;
border-radius: 3px;
cursor: pointer;
}
```
最后是 JavaScript 文件 `script.js`:
```javascript
function login() {
var username = document.getElementById("username").value;
var password = document.getElementById("password").value;
// 发送登录请求到后端
fetch('/login', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({
username: username,
password: password
})
})
.then(response => response.json())
.then(data => {
if (data.success) {
alert("登录成功!");
} else {
alert("登录失败:" + data.message);
}
})
.catch(error => console.error(error));
}
```
在后端使用 Node.js 和 Express 框架,读取本地 Excel 数据使用了 `xlsx` 库,以下是后端代码:
```javascript
const express = require('express');
const app = express();
const xlsx = require('xlsx');
const bodyParser = require('body-parser');
const workbook = xlsx.readFile('users.xlsx');
const worksheet = workbook.Sheets['Sheet1'];
const users = xlsx.utils.sheet_to_json(worksheet);
app.use(bodyParser.json());
app.post('/login', (req, res) => {
const username = req.body.username;
const password = req.body.password;
const user = users.find(u => u.username === username && u.password === password);
if (user) {
res.json({ success: true });
} else {
res.json({ success: false, message: '用户名或密码错误' });
}
});
app.listen(3000, () => console.log('Server started on port 3000'));
```
这个示例代码使用了简单的用户名和密码验证,可以根据实际需求进行修改。需要注意的是,这个示例代码并没有对密码进行加密处理,实际应用中需要使用加密算法对密码进行保护。同时,这个示例代码也没有使用 HTTPS 协议,实际应用中需要使用 HTTPS 协议来保证通信的安全性。
阅读全文
相关推荐
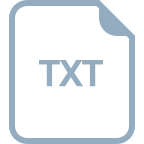

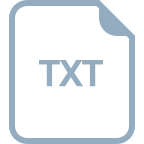
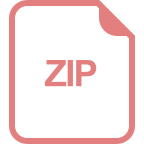
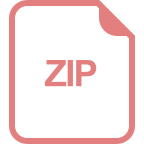
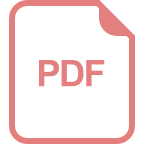
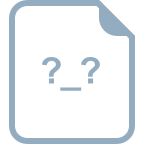

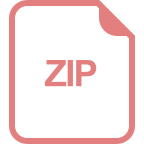
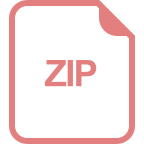
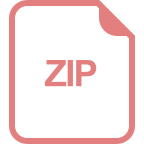
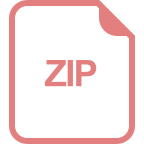
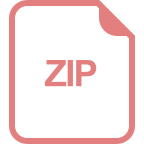
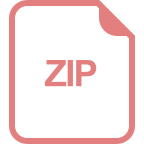
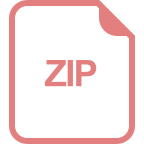
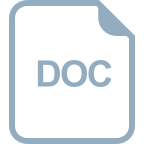
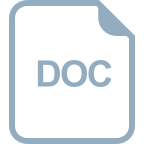