#include <iostream> #include <ctime> using namespace std; struct userNode { int key; bool sex; int birthday; struct userNode* next = NULL; }; userNode* HashTable[298]; int Hash(int key)//散列函数 { int res = 0; while (key) { res += key % 100; key /= 100; } return res; } userNode* Login(int key)//查找 { int afterHash = Hash(key); userNode* p = HashTable[afterHash]; while (p && p->key != key) { p = p->next; } if (p && (p->key == key)) { return p; } else { return NULL; } return NULL; } int Register(userNode* newUser)//插入 { int afterHash = Hash(newUser->key); userNode* p = HashTable[afterHash]; while (p) { p = p->next; } newUser->next = HashTable[afterHash]; HashTable[afterHash] = newUser; return 0; } int main() { userNode* nowTmp; int tmp; while (1) { system("cls"); cout << "请输入你的PP号:" << endl; tmp = 0; while (tmp < 100000 || tmp>999999) { cin >> tmp; } nowTmp = Login(tmp); if (nowTmp) { system("cls"); cout << "-------------------------------------------" << endl << "| 登录成功! |" << endl << "| qq号:" << nowTmp->key << " |" << endl << "| 性别:" << (nowTmp->sex ? "男" : "女") << " |" << endl << "| 生日:" << nowTmp->birthday << " |" << endl << "-------------------------------------------" << endl; system("pause"); } else { // 自动注册 userNode *p; p->key = tmp; p->birthday = 2002 + rand() % 21; p->birthday *= 100; p->birthday += 1 + rand() % 12; p->birthday *= 100; p->birthday += 1 + rand() % 28; p->sex = rand() % 2; Register(p); cout << "这个PP号还没注册!帮你注册了!请重新登录!" << endl; system("pause"); } } return 0; }逐行注释
时间: 2024-01-04 21:04:57 浏览: 21
#include <iostream>
#include <ctime> //用于生成随机数的时间戳
using namespace std;
struct userNode { //定义用户信息结构体
int key; //用户的PP号
bool sex; //用户性别,0为女,1为男
int birthday; //用户生日,格式为YYYYMMDD
struct userNode* next = NULL; //下一个用户信息结构体的指针,默认为NULL
};
userNode* HashTable[298]; //哈希表,用于存储用户信息
int Hash(int key)//散列函数,根据PP号生成一个哈希值
{
int res = 0;
while (key) {
res += key % 100; //取PP号的后两位相加
key /= 100; //除以100,向前移动两位
}
return res; //返回哈希值
}
userNode* Login(int key)//查找用户信息
{
int afterHash = Hash(key); //根据PP号生成哈希值
userNode* p = HashTable[afterHash]; //从哈希表中取出对应位置的结构体指针
while (p && p->key != key) { //如果结构体指针存在且PP号不符合
p = p->next; //继续向下一个结构体指针移动
}
if (p && (p->key == key)) { //如果结构体指针存在,且PP号匹配
return p; //返回对应的结构体指针
} else { //否则返回NULL
return NULL;
}
return NULL; //无论如何都会返回NULL
}
int Register(userNode* newUser)//插入新用户信息
{
int afterHash = Hash(newUser->key); //根据PP号生成哈希值
userNode* p = HashTable[afterHash]; //从哈希表中取出对应位置的结构体指针
while (p) { //如果结构体指针存在
p = p->next; //继续向下一个结构体指针移动
}
newUser->next = HashTable[afterHash]; //新用户信息结构体的下一个指针指向原来的结构体指针
HashTable[afterHash] = newUser; //将新用户信息结构体的指针存入哈希表中
return 0; //插入成功,返回0
}
int main() {
userNode* nowTmp; //定义当前用户信息结构体指针
int tmp; //定义临时变量
while (1) { //循环执行
system("cls"); //清屏
cout << "请输入你的PP号:" << endl;
tmp = 0;
while (tmp < 100000 || tmp>999999) { //如果输入的PP号不符合条件
cin >> tmp; //重新输入
}
nowTmp = Login(tmp); //根据输入的PP号查找对应的用户信息
if (nowTmp) { //如果用户信息存在
system("cls"); //清屏
cout << "-------------------------------------------" << endl
<< "| 登录成功! |" << endl
<< "| qq号:" << nowTmp->key << " |" << endl
<< "| 性别:" << (nowTmp->sex ? "男" : "女") << " |" << endl
<< "| 生日:" << nowTmp->birthday << " |" << endl
<< "-------------------------------------------" << endl;
system("pause"); //暂停程序,等待用户操作
} else { //如果用户信息不存在
userNode *p; //定义新的用户信息结构体指针
p->key = tmp; //设置PP号
p->birthday = 2002 + rand() % 21; //随机生成生日年份,范围在2002到2022之间
p->birthday *= 100; //将年份左移两位
p->birthday += 1 + rand() % 12; //随机生成生日月份,范围在1到12之间
p->birthday *= 100; //将月份左移两位
p->birthday += 1 + rand() % 28; //随机生成生日日份,范围在1到28之间
p->sex = rand() % 2; //随机生成性别,0为女,1为男
Register(p); //将新用户信息存入哈希表中
cout << "这个PP号还没注册!帮你注册了!请重新登录!" << endl; //提示用户已经自动注册
system("pause"); //暂停程序,等待用户操作
}
}
return 0;
}
相关推荐
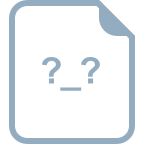














