beautifulsoup爬取生成excel
时间: 2023-09-18 12:06:31 浏览: 123
### 回答1:
可以使用Python中的BeautifulSoup库来爬取网页数据,并使用pandas库将数据存储到Excel文件中。
首先,需要安装BeautifulSoup和pandas库:
```
pip install beautifulsoup4
pip install pandas
```
然后,可以使用以下代码来爬取网页数据并将其存储到Excel文件中:
```python
import requests
from bs4 import BeautifulSoup
import pandas as pd
# 发送请求获取网页内容
url = 'https://www.example.com'
response = requests.get(url)
html = response.content
# 使用BeautifulSoup解析网页内容
soup = BeautifulSoup(html, 'html.parser')
# 获取需要的数据
data = []
table = soup.find('table')
rows = table.find_all('tr')
for row in rows:
cols = row.find_all('td')
cols = [col.text.strip() for col in cols]
data.append(cols)
# 将数据存储到Excel文件中
df = pd.DataFrame(data)
df.to_excel('data.xlsx', index=False, header=False)
```
这段代码会将网页中的表格数据爬取下来,并存储到名为"data.xlsx"的Excel文件中。可以根据实际情况修改代码中的网址和数据解析方式。
### 回答2:
使用BeautifulSoup进行网页爬取,并将数据保存为Excel文件是一种常见且方便的方法。下面是一个示例代码:
首先,我们需要导入所需的库:
```python
from bs4 import BeautifulSoup
import requests
import pandas as pd
```
然后,我们可以使用Requests库获取要爬取的网页内容:
```python
url = '要爬取的网页地址'
response = requests.get(url)
```
接下来,我们可以使用BeautifulSoup库来解析网页内容并提取所需的数据:
```python
soup = BeautifulSoup(response.text, 'html.parser')
# 根据网页结构和需要的数据,使用BeautifulSoup提取相应的数据
data = []
# 示例:获取网页中所有的标题
titles = soup.find_all('h1')
for title in titles:
data.append(title.text)
```
最后,我们可以使用Pandas库将数据保存为Excel文件:
```python
df = pd.DataFrame(data, columns=['标题名称'])
# 保存为Excel文件
df.to_excel('文件名.xlsx', index=False)
```
以上就是使用BeautifulSoup爬取网页并生成Excel文件的基本步骤。根据具体的网页结构和需要提取的数据,可以进行相应的调整和扩展。
### 回答3:
BeautifulSoup是一个Python库,用于从HTML或XML文档中提取数据。通过使用BeautifulSoup爬取数据后,我们可以使用其他库,如Pandas和Openpyxl,将数据存储到Excel文件中。
首先,我们需要安装BeautifulSoup库。在命令提示符或终端中输入以下命令:
pip install beautifulsoup4
接下来,我们需要导入相应的库。在Python脚本的开头添加以下行:
from bs4 import BeautifulSoup
import pandas as pd
from openpyxl import Workbook
然后,我们需要使用BeautifulSoup来解析HTML或XML文档。我们可以使用requests库进行网页请求,并将其传递给BeautifulSoup来解析数据。下面是一个示例:
import requests
url = "网页的URL"
response = requests.get(url)
soup = BeautifulSoup(response.text, "html.parser")
接下来,我们需要提取想要存储到Excel的数据。我们可以使用BeautifulSoup的查找和选择器功能来定位特定的HTML标签或CSS选择器。以下是一个示例:
data = []
table = soup.find("table") # 假设要提取HTML文档中的表格数据
rows = table.find_all("tr") # 查找所有行
for row in rows:
cells = row.find_all("td") # 查找行中的所有单元格
row_data = []
for cell in cells:
row_data.append(cell.text) # 提取单元格的文本数据
data.append(row_data) # 将每一行的数据添加到data列表中
接下来,我们可以使用Pandas库将数据转换为DataFrame对象,并使用openpyxl库将DataFrame写入Excel文件。以下是一个示例:
df = pd.DataFrame(data)
df.columns = ["列名1", "列名2", ...] # 设置DataFrame的列名
# 创建一个Workbook对象
wb = Workbook()
sheet = wb.active
# 将DataFrame的数据写入工作表中
for r in dataframe_to_rows(df, index=False, header=True):
sheet.append(r)
# 保存Excel文件
wb.save("文件名.xlsx")
这样,我们就用BeautifulSoup爬取数据并将其存储到Excel文件中了。不过,请注意在实际应用中可能需要根据具体情况进行调整和优化。
阅读全文
相关推荐
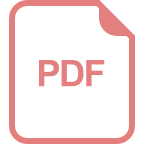
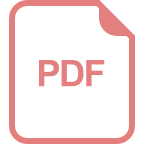
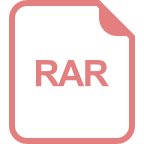

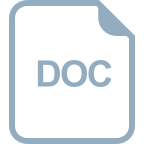



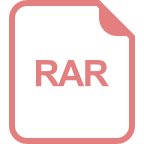










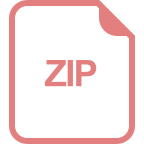