vue项目oppo商城分类页中的点击切换数据
时间: 2024-03-13 10:42:32 浏览: 32
在Vue项目中,实现点击切换数据的功能可以使用`v-for`指令和`v-bind`指令。
假设我们的数据结构如下:
```
{
"categories": [
{
"id": 1,
"name": "手机",
"products": [
{
"id": 101,
"name": "OPPO Find X3 Pro",
"price": 5999
},
{
"id": 102,
"name": "OPPO Reno5 Pro+",
"price": 3999
}
]
},
{
"id": 2,
"name": "电视",
"products": [
{
"id": 201,
"name": "OPPO TV S1",
"price": 2999
},
{
"id": 202,
"name": "OPPO TV R1",
"price": 1999
}
]
}
]
}
```
其中,`categories`是一个数组,包含多个分类,每个分类又包含一个`id`、一个`name`和一个`products`数组,`products`数组中包含多个商品,每个商品又包含一个`id`、一个`name`和一个`price`。
我们可以使用一个`categories`数组来存储这些数据,然后使用一个`selectedCategoryId`变量来记录当前选中的分类的`id`。在模板中,我们可以使用`v-for`指令来循环渲染分类,使用`v-bind`指令来动态绑定类名,以实现点击切换数据的效果。
具体代码如下:
HTML代码:
```
<template>
<div class="categories">
<div class="category" v-for="category in categories" :class="{ active: category.id === selectedCategoryId }" @click="selectCategory(category.id)">
{{ category.name }}
</div>
<div class="products">
<div class="product" v-for="product in selectedCategory.products">
{{ product.name }} - {{ product.price }}
</div>
</div>
</div>
</template>
```
CSS代码:
```
.category {
display: inline-block;
padding: 10px;
margin-right: 10px;
border-radius: 5px;
cursor: pointer;
}
.category.active {
background-color: #f60;
color: #fff;
}
.products {
margin-top: 20px;
}
.product {
margin-bottom: 10px;
}
```
JavaScript代码:
```
export default {
data() {
return {
categories: [
{
id: 1,
name: "手机",
products: [
{
id: 101,
name: "OPPO Find X3 Pro",
price: 5999
},
{
id: 102,
name: "OPPO Reno5 Pro+",
price: 3999
}
]
},
{
id: 2,
name: "电视",
products: [
{
id: 201,
name: "OPPO TV S1",
price: 2999
},
{
id: 202,
name: "OPPO TV R1",
price: 1999
}
]
}
],
selectedCategoryId: 1
};
},
methods: {
selectCategory(categoryId) {
this.selectedCategoryId = categoryId;
}
},
computed: {
selectedCategory() {
return this.categories.find(category => category.id === this.selectedCategoryId);
}
}
};
```
在上面的代码中,我们使用了一个`selectCategory`方法来更新`selectedCategoryId`变量,以实现点击切换分类的效果。同时,我们使用了一个`selectedCategory`计算属性来获取当前选中的分类对象,以便在模板中渲染该分类下的商品。
相关推荐
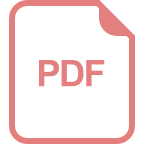
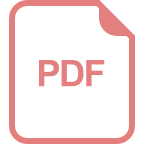














