java 组合模式 代码例子
时间: 2023-05-21 16:01:50 浏览: 68
以下是一个简单的 Java 组合模式的代码例子:
```
public abstract class Component {
protected String name;
public Component(String name) {
this.name = name;
}
public abstract void add(Component component);
public abstract void remove(Component component);
public abstract void display(int depth);
}
public class Leaf extends Component {
public Leaf(String name) {
super(name);
}
public void add(Component component) {
System.out.println("Cannot add to a leaf");
}
public void remove(Component component) {
System.out.println("Cannot remove from a leaf");
}
public void display(int depth) {
System.out.println("-".repeat(depth) + name);
}
}
public class Composite extends Component {
private List<Component> children = new ArrayList<>();
public Composite(String name) {
super(name);
}
public void add(Component component) {
children.add(component);
}
public void remove(Component component) {
children.remove(component);
}
public void display(int depth) {
System.out.println("-".repeat(depth) + name);
for (Component component : children) {
component.display(depth + 2);
}
}
}
// Usage example
public class Main {
public static void main(String[] args) {
Composite root = new Composite("root");
root.add(new Leaf("Leaf A"));
root.add(new Leaf("Leaf B"));
Composite composite = new Composite("Composite X");
composite.add(new Leaf("Leaf XA"));
composite.add(new Leaf("Leaf XB"));
root.add(composite);
root.display(1);
}
}
```
这个例子中,`Component` 是组合中的抽象类,`Leaf` 是叶子节点,`Composite` 是组合节点。`Composite` 中包含了一个 `List` 来存储它的子节点。在 `Composite` 中,`add` 方法和 `remove` 方法用来添加和删除子节点,`display` 方法用来显示节点的名称和它的子节点。在 `Leaf` 中,`add` 和 `remove` 方法都会输出错误信息,因为叶子节点没有子节点。在 `Main` 类中,我们创建了一个根节点 `root`,并向它添加了两个叶子节点和一个组合节点。最后,我们调用 `root.display(1)` 来显示整个树形结构。
相关推荐
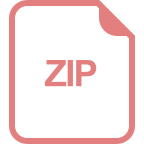














