用js写一个卷积神经网络
时间: 2023-05-12 13:06:40 浏览: 45
当然可以,以下是一个简单的卷积神经网络的示例代码:
```javascript
// 定义卷积层
class ConvLayer {
constructor(inputDepth, inputWidth, inputHeight, filterSize, filterNum, stride, padding) {
this.inputDepth = inputDepth;
this.inputWidth = inputWidth;
this.inputHeight = inputHeight;
this.filterSize = filterSize;
this.filterNum = filterNum;
this.stride = stride;
this.padding = padding;
this.outputWidth = Math.floor((inputWidth - filterSize + 2 * padding) / stride) + 1;
this.outputHeight = Math.floor((inputHeight - filterSize + 2 * padding) / stride) + 1;
this.outputDepth = filterNum;
this.filters = [];
for (let i = 0; i < filterNum; i++) {
this.filters.push(new Filter(filterSize, inputDepth));
}
}
forward(input) {
this.input = input;
let output = new Array(this.outputWidth * this.outputHeight * this.outputDepth);
for (let i = 0; i < this.outputDepth; i++) {
let f = this.filters[i];
let y = 0;
for (let j = 0; j < this.outputHeight; j++) {
let x = 0;
for (let k = 0; k < this.outputWidth; k++) {
let dot = 0;
for (let l = 0; l < this.inputDepth; l++) {
for (let m = 0; m < f.size; m++) {
for (let n = 0; n < f.size; n++) {
let inputIndex = (j * this.stride + m) * this.inputWidth * this.inputDepth +
(k * this.stride + n) * this.inputDepth + l;
let filterIndex = m * f.size * this.inputDepth + n * this.inputDepth + l;
dot += input[inputIndex] * f.weights[filterIndex];
}
}
}
output[y * this.outputWidth + x] = dot + f.bias;
x++;
}
y++;
}
}
return output;
}
}
// 定义过滤器
class Filter {
constructor(size, depth) {
this.size = size;
this.weights = new Array(size * size * depth);
this.bias = 0;
for (let i = 0; i < this.weights.length; i++) {
this.weights[i] = Math.random() - 0.5;
}
}
}
// 定义全连接层
class FCLayer {
constructor(inputSize, outputSize) {
this.inputSize = inputSize;
this.outputSize = outputSize;
this.weights = new Array(inputSize * outputSize);
this.bias = new Array(outputSize);
for (let i = 0; i < this.weights.length; i++) {
this.weights[i] = Math.random() - 0.5;
}
for (let i = 0; i < this.bias.length; i++) {
this.bias[i] = Math.random() - 0.5;
}
}
forward(input) {
this.input = input;
let output = new Array(this.outputSize);
for (let i = 0; i < this.outputSize; i++) {
let dot = 0;
for (let j = 0; j < this.inputSize; j++) {
dot += input[j] * this.weights[j * this.outputSize +
相关推荐
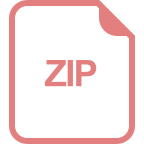
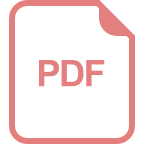
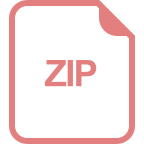














