用C++写出单链表的插入
时间: 2024-03-23 12:15:11 浏览: 26
节点的代码。
以下是示例代码:
```c
// 定义节点结构体
typedef struct ListNode {
int data; // 节点数据
struct ListNode *next; // 指向下一个节点的指针
} ListNode;
// 定义单链表头指针
ListNode *head = NULL;
// 定义单链表节点插入函数
void insertNode(int data)
{
// 创建新节点
ListNode *newNode = (ListNode*)malloc(sizeof(ListNode));
newNode->data = data;
newNode->next = NULL;
// 如果链表为空,将新节点作为头节点
if (head == NULL) {
head = newNode;
return;
}
// 插入新节点
ListNode *current = head;
while (current->next != NULL) {
current = current->next;
}
current->next = newNode;
}
```
注意,这是单链表的末尾插入节点的示例代码,如果需要在链表中间或头部插入节点,需要做相应的修改。
相关问题
用c++单链表写出学生管理系统
学生管理系统的c语言单链表实现可以建立如下:定义结构体:struct Student{
char name[20]; //姓名
int age; //年龄
char sex[4]; //性别
struct Student *next; //指向下一个学生的指针
};创建链表:struct Student *CreateLink(){
struct Student *head;
struct Student *p1,*p2;
int n=0;
p1=p2=(struct Student *)malloc(sizeof(struct Student));
scanf("%s %d %s",p1->name,&p1->age,p1->sex);
p1->next=NULL;
head=NULL;
while(p1->name[0]!='0'){
n++;
if(n==1) head=p1;
else p2->next=p1;
p2=p1;
p1=(struct Student *)malloc(sizeof(struct Student));
scanf("%s %d %s",p1->name,&p1->age,p1->sex);
p1->next=NULL;
}
return(head);
}插入节点:struct Student *InsertNode(struct Student *head,struct Student *stud){
struct Student *p0,*p1,*p2;
p1=head;
p0=stud;
if(head==NULL){
head=p0;
p0->next=NULL;
}
else{
while((p0->age > p1->age) && (p1->next!=NULL)){
p2=p1;
p1=p1->next;
}
if(p0->age <= p1->age){
if(head==p1) head=p0;
else p2->next=p0;
p0->next=p1;
}
else{
p1->next=p0;
p0->next=NULL;
}
}
return(head);
}查找节点:struct Student *FindNode(struct Student *head,char *name){
struct Student *p;
p=head;
if(head==NULL) return(NULL);
else{
while(strcmp(name,p->name)!=0){
p=p->next;
if(p==NULL) return(NULL);
}
return(p);
}
}删除节点:struct Student *DeleteNode(struct Student *head,char *name){
struct Student *p1,*p2;
if(head==NULL) return(head);
p1=head;
while(strcmp(name,p1->name)!=0){
p2=p1;
p1=p1->next;
if(p1==NULL) return(head);
}
if(p1==head) head=p1->next;
else p2->next=p1->next;
free(p1);
return(head);
}
写出单链表的完整操作代码
下面是单链表的完整操作代码,包括创建、插入、删除、查找、遍历等基本操作:
```C++
#include <iostream>
using namespace std;
// 定义链表节点结构体
struct ListNode {
int val;
ListNode* next;
ListNode(int x) : val(x), next(NULL) {}
};
// 创建链表
ListNode* createList(int arr[], int n) {
if (n == 0) {
return NULL;
}
ListNode* head = new ListNode(arr[0]);
ListNode* cur = head;
for (int i = 1; i < n; i++) {
cur->next = new ListNode(arr[i]);
cur = cur->next;
}
return head;
}
// 插入节点
void insertNode(ListNode* head, int val) {
ListNode* node = new ListNode(val);
ListNode* cur = head;
while (cur->next != NULL) {
cur = cur->next;
}
cur->next = node;
}
// 删除节点
void deleteNode(ListNode* head, int val) {
ListNode* cur = head;
while (cur->next != NULL && cur->next->val != val) {
cur = cur->next;
}
if (cur->next != NULL) {
ListNode* temp = cur->next;
cur->next = cur->next->next;
delete temp;
}
}
// 查找节点
ListNode* findNode(ListNode* head, int val) {
ListNode* cur = head;
while (cur != NULL && cur->val != val) {
cur = cur->next;
}
return cur;
}
// 遍历链表
void traverseList(ListNode* head) {
ListNode* cur = head;
while (cur != NULL) {
cout << cur->val << " ";
cur = cur->next;
}
cout << endl;
}
int main() {
int arr[] = {1, 2, 3, 4};
ListNode* head = createList(arr, 4);
// 插入节点
insertNode(head, 5);
traverseList(head);
// 删除节点
deleteNode(head, 3);
traverseList(head);
// 查找节点
ListNode* node = findNode(head, 2);
if (node != NULL) {
cout << "Find node: " << node->val << endl;
} else {
cout << "Not found!" << endl;
}
return 0;
}
```
上面的代码中,我们定义了一个链表节点结构体 `ListNode`,包含一个整数值 `val` 和一个指向下一个节点的指针 `next`。然后我们实现了创建链表、插入节点、删除节点、查找节点、遍历链表等基本操作。在 `main` 函数中,我们创建了一个包含 {1, 2, 3, 4} 的链表,然后测试了插入、删除、查找、遍历等操作。
相关推荐
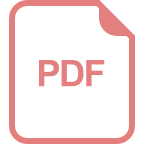









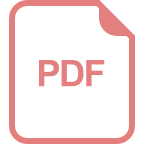
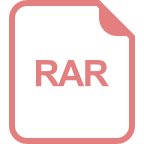
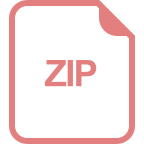
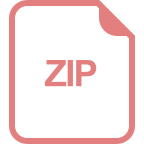