C++使用openssl实现字符串的Base64编码
时间: 2024-02-21 15:00:37 浏览: 174
可以使用OpenSSL库中的函数来实现字符串的Base64编码,下面是一个使用C++和OpenSSL库实现字符串的Base64编码的例子:
```c++
#include <openssl/bio.h>
#include <openssl/evp.h>
#include <iostream>
#include <cstring>
int main() {
// 要编码的字符串
std::string str = "Hello, world!";
// 初始化BIO
BIO *bio, *b64;
b64 = BIO_new(BIO_f_base64());
bio = BIO_new(BIO_s_mem());
bio = BIO_push(b64, bio);
// 编码
BIO_write(bio, str.c_str(), static_cast<int>(str.length()));
BIO_flush(bio);
// 获取编码结果
char *enc_data;
long len = BIO_get_mem_data(bio, &enc_data);
std::string encoded_str(enc_data, len);
// 清理
BIO_free_all(bio);
// 输出编码结果
std::cout << "Encoded string: " << encoded_str << std::endl;
return 0;
}
```
在上面的例子中,我们使用了OpenSSL库中的BIO函数来进行Base64编码。首先,我们初始化了一个BIO链,将BIO_f_base64()作为编码器插入到BIO_s_mem()中,然后将我们要编码的字符串写入BIO并刷新,最后通过BIO_get_mem_data()获取编码结果。
需要注意的是,使用完BIO后需要使用BIO_free_all()来清理BIO。
阅读全文
相关推荐
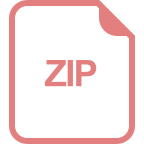

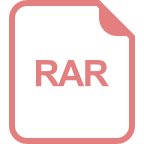




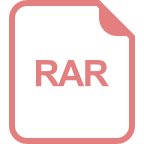
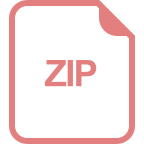
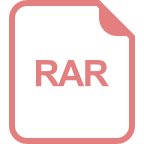
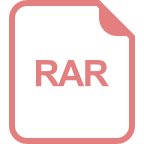
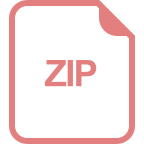
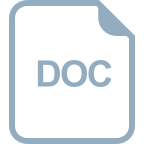



