c++ openssl rsa 字符串公私钥加解密示例代码
时间: 2024-10-23 18:20:34 浏览: 20
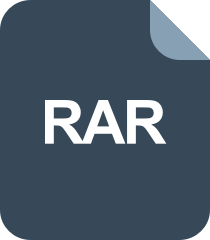
c++ 加密字符串 工具 代码 工具一键生成
在C++中使用OpenSSL库处理RSA加密解密,你需要包含相关的头文件,并利用`BIO`和`RSA`结构来操作公钥和私钥。下面是一个简单的示例,展示了如何生成密钥对、加密字符串、然后用私钥解密:
```cpp
#include <openssl/bio.h>
#include <openssl/rsa.h>
#include <openssl/pem.h>
#include <string>
// 生成RSA密钥对
std::string generate_key_pair() {
RSA *rsa = RSA_new();
BIGNUM *e = BN_new(), *n = BN_new();
// 生成大素数n和e(通常e=65537)
BN_rand_range(n, RSA_NBITS);
BN_set_word(e, RSA_F4);
// 创建RSA结构并设置参数
RSA_generate_key_ex(rsa, RSA modulus bits, e, n, NULL, NULL);
// 将公钥和私钥转换为PEM格式的字符串
BIO* bio_pub = BIO_new(BIO_s_mem());
BIO* bio_priv = BIO_new(BIO_s_mem());
PEM_write_bio_RSA_PUBKEY(bio_pub, rsa);
PEM_write_bio_RSAPrivateKey(bio_priv, rsa, NULL, NULL);
std::string pub_str = BIO_get_mem_data(bio_pub), priv_str = BIO_get_mem_data(bio_priv);
RSA_free(rsa);
BN_free(e);
BN_free(n);
BIO_free_all(bio_pub);
BIO_free_all(bio_priv);
return "Public Key:\n" + pub_str + "\nPrivate Key:\n" + priv_str;
}
// 加密函数
std::string encrypt(const std::string& plaintext, const std::string& public_key) {
RSA *rsaPub = RSA_new();
rsaPub = PEM_read_RSA_PUBKEY(RSA_new(), (char*)public_key.c_str(), NULL, NULL);
BIO* bio_in = BIO_new(BIO_f_base64());
BIO* bio_out = BIO_new(BIO_s_mem());
BIO_write(bio_in, plaintext.c_str(), plaintext.length());
BIO_push(bio_out, bio_in);
RSA_public_encrypt(plaintext.size(), BIO_get_mem_ptr(bio_out), rsaPub, RSA_PKCS1_OAEP_PADDING);
std::string ciphertext = BIO_get_mem_data(bio_out);
BIO_free_all(bio_out);
RSA_free(rsaPub);
return ciphertext;
}
// 解密函数
std::string decrypt(const std::string& ciphertext, const std::string& private_key) {
RSA *rsaPri = RSA_new();
rsaPri = PEM_read_PrivateKey(RSA_new(), (char*)private_key.c_str(), NULL, NULL);
BIO* bio_in = BIO_new(BIO_f_base64());
BIO* bio_out = BIO_new(BIO_s_mem());
BIO_write(bio_in, ciphertext.c_str(), ciphertext.length());
BIO_push(bio_out, bio_in);
RSA_private_decrypt(ciphertext.size(), BIO_get_mem_ptr(bio_in), rsaPri, RSA_PKCS1_OAEP_PADDING);
std::string plaintext = BIO_get_mem_data(bio_out);
BIO_free_all(bio_out);
RSA_free(rsaPri);
return plaintext;
}
int main() {
auto key_pairs = generate_key_pair();
std::cout << "Keys generated.\n";
std::string encrypted_text = encrypt("Hello, World!", key_pairs.substr(key_pairs.find("\n")+1));
std::cout << "Text encrypted.\n";
std::string decrypted_text = decrypt(encrypted_text, key_pairs.substr(0, key_pairs.find("\n")));
std::cout << "Decrypted text: " << decrypted_text << std::endl;
return 0;
}
```
注意:这个示例仅供参考,实际应用中你需要处理错误检查和异常处理。此外,为了安全存储和传输私钥,建议将其保存在安全的地方,而不是明文显示。
阅读全文
相关推荐
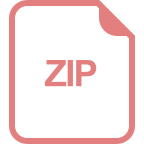
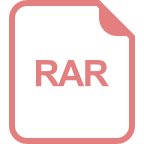



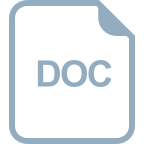




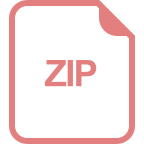
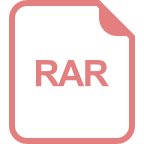
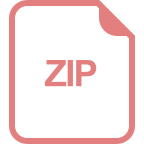
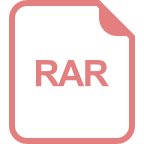



