c++ openssl rsa从字符串中读取公私钥加解密 示例代码
时间: 2024-10-23 08:20:34 浏览: 37
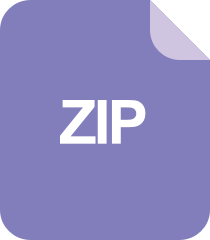
c++字符串简单加密.zip_C 字符串 加密_C 简单加密_C++_DEMO_字符串加密
在C++中,使用OpenSSL库处理RSA加密解密操作通常涉及`openssl PEM_read_bio_*`系列函数来读取PEM格式的公钥和私钥,以及`RSA_public_encrypt`和`RSA_private_decrypt`函数来进行数据的加解密。下面是一个简单的示例代码片段,展示如何从字符串中读取公钥和私钥,并进行加解密:
```cpp
#include <openssl/pem.h>
#include <openssl/rsa.h>
std::string privateKeyPEM = "-----BEGIN RSA PRIVATE KEY-----\n"
"MIIEowIBAAKCAQEAwJH6V7q4+... (私钥内容省略)\n"
"-----END RSA PRIVATE KEY-----";
std::string publicKeyPEM = "-----BEGIN PUBLIC KEY-----\n"
"MIICIjANBgkqhkiG9w0BAQEFAAOCAg8AMIICCgKCAgEAtiFhj... (公钥内容省略)\n"
"-----END PUBLIC KEY-----";
// 解析私钥
RSA *pkeyPrivate;
BIO *bioPrivKey = BIO_new_mem_buf((unsigned char*)privateKeyPEM.c_str(), -1);
if (!pkeyPrivate = PEM_read_bio_RSAPrivateKey(bioPrivKey, NULL, NULL, NULL)) {
// 错误处理...
}
// 解析公钥
RSA *pkeyPublic;
BIO *bioPubKey = BIO_new_mem_buf((unsigned char*)publicKeyPEM.c_str(), -1);
if (!pkeyPublic = PEM_read_bio_RSA_PUBKEY(bioPubKey, NULL, NULL, NULL)) {
// 错误处理...
}
// 加密示例(使用公钥)
const unsigned char plaintext[] = "Hello, world!";
size_t plaintextLen = strlen(plaintext);
unsigned char ciphertext[1024];
size_t ciphertextLen = 0;
int result = RSA_public_encrypt(plaintextLen, plaintext, ciphertext, pkeyPublic, RSA_PKCS1_OAEP_PADDING);
if (result > 0) {
std::cout << "Encrypted message: ";
for (size_t i = 0; i < ciphertextLen; ++i) {
printf("%02X ", ciphertext[i]);
}
std::cout << std::endl;
} else {
// 错误处理...
}
// 解密示例(使用私钥)
unsigned char decryptedData[ciphertextLen + 1]; // 加1是为了容纳额外的null终止符
result = RSA_private_decrypt(ciphertextLen, ciphertext, decryptedData, pkeyPrivate, RSA_PKCS1_OAEP_PADDING);
if (result >= 0 && decryptedData[result] == '\0') { // 检查解密是否成功且有正确的终止符
std::cout << "Decrypted message: " << decryptedData << std::endl;
} else {
// 错误处理...
}
// 清理资源
BIO_free_all(bioPrivKey);
BIO_free_all(bioPubKey);
RSA_free(pkeyPrivate);
RSA_free(pkeyPublic);
阅读全文
相关推荐
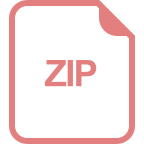
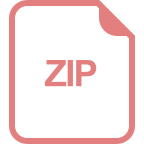




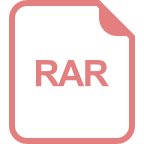
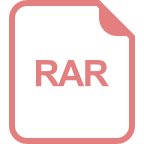
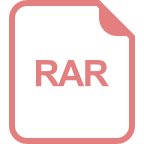
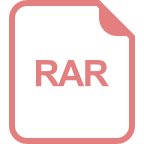
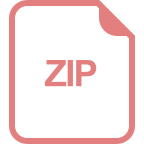
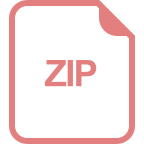
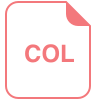




