c++ openssl rsa从字符串中读取公私钥加解密
时间: 2024-10-23 15:20:37 浏览: 42
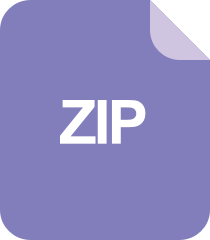
c++字符串简单加密.zip_C 字符串 加密_C 简单加密_C++_DEMO_字符串加密
在C++中使用OpenSSL库操作RSA加密解密,首先需要包含必要的头文件并初始化OpenSSL上下文。如果你想要从字符串中读取公钥或私钥,你可以按照以下步骤操作:
1. 包含必要的头文件:
```cpp
#include <openssl/pem.h>
#include <openssl/rsa.h>
```
2. 使用`PEM_read_bio_X509()`函数读取公钥(PEM编码),如果需要私钥,则使用`PEM_read_PrivateKey()`:
```cpp
std::string publicKeyPEM = "-----BEGIN PUBLIC KEY----- ... -----END PUBLIC KEY-----"; // 公钥字符串
RSA* rsaPubKey = PEM_read_RSA_PUBKEY(RSA_METHOD(), reinterpret_cast<unsigned char*>(publicKeyPEM.c_str()), nullptr, nullptr);
// 私钥类似:
std::string privateKeyPEM = "-- ... -----END RSA PRIVATE KEY-----";
RSA* rsaPrivKey = PEM_read_RSAPrivateKey(RSA_METHOD(), reinterpret_cast<unsigned char*>(privateKeyPEM.c_str()), nullptr, nullptr);
```
注意:这里的`nullptr`表示密码为空,如果你的私钥有密码保护,你需要提供正确的密码。
3. 加密数据:
```cpp
unsigned char encryptedData[1024];
int encryptedLength;
std::string dataToEncrypt = "plaintext";
if (rsaPubKey) {
int result = RSA_public_encrypt(strlen(dataToEncrypt), reinterpret_cast<const unsigned char*>(dataToEncrypt.c_str()), encryptedData, rsaPubKey, RSA_PKCS1_OAEP_PADDING);
if (result > 0) {
encryptedLength = result;
// 然后将加密后的数据存储到某个地方
}
}
```
4. 解密数据:
```cpp
std::vector<unsigned char> decryptedData(encryptedLength);
if (rsaPrivKey) {
int decryptedResult = RSA_private_decrypt(encryptedLength, encryptedData, &decryptedData[0], rsaPrivKey, RSA_PKCS1_OAEP_PADDING);
if (decryptedResult == -1 && SSL_get_error(rsaPrivKey->meth, decryptedResult) == SSL_ERROR_DECRYPT_ERROR) {
std::cerr << "Decryption failed due to padding error." << std::endl;
} else if (decryptedResult == -1) {
std::cerr << "Decryption failed with other errors." << std::endl;
} else {
std::string decryptedString(reinterpret_cast<char*>(&decryptedData[0]), decryptedResult);
// 显示或处理解密后的数据
}
}
```
记得在使用完后释放内存和关闭资源:
```cpp
RSA_free(rsaPubKey);
RSA_free(rsaPrivKey);
```
阅读全文
相关推荐
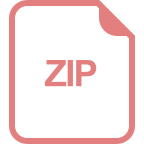
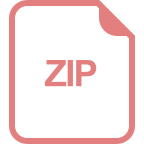















