1、C++实现RSA加密算法 通过自己编写的RSA算法实现对文档进行加解密、数字签名方法
时间: 2024-10-20 22:17:16 浏览: 78
在C++中实现RSA加密算法,首先需要了解其基本原理:RSA是一种非对称加密算法,它使用两个密钥——公钥和私钥来进行信息的加密和解密。公钥可以公开,而私钥必须保密。
以下是简单的步骤和代码概述:
1. **生成密钥对**:
- 使用大素数作为模数n,选择两个互质的整数e和d,其中e用于加密(欧拉函数的逆),d用于解密。
```cpp
int p, q;
BigInteger n = modProduct(p, q); // 生成大素数n
BigInteger phi(n);
BigInteger e = generatePublicExponent(); // 非极大公约数
BigInteger d = inverse(e, phi); // 私钥
```
2. **公钥和私钥的表示**:
- 可以使用`BigInteger`库来存储大整数,并将它们保存为字符串形式。
```cpp
std::string publicKey("e=" + e.toString() + ", n=" + n.toString());
std::string privateKey("d=" + d.toString() + ", n=" + n.toString());
```
3. **加密和解密**:
- 加密时使用公钥(e和n),计算ciphertext = message^e % n;
- 解密时使用私钥(d和n),计算plaintext = ciphertext^d % n。
```cpp
std::string ciphertext = encrypt(message, publicKey, e, n);
std::string plaintext = decrypt(ciphertext, privateKey, d, n);
```
4. **数字签名**(利用私钥):
- 对原始数据使用哈希函数得到消息摘要,然后对摘要进行加密,生成签名。
```cpp
std::string signature = sign(message, privateKey, hashFunction(plaintext));
```
- 验证签名时,检查接收方能否使用接收者的公钥成功解密签名并还原哈希值。
请注意,这只是一个简化的概述,实际应用中需要考虑安全性、大整数运算效率以及错误处理等问题。此外,开源库如 OpenSSL 或 Botan 等提供了完整的 RSA 实现,可以直接使用。
阅读全文
相关推荐
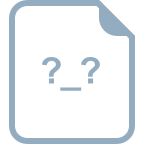
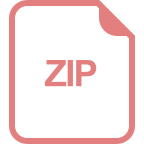
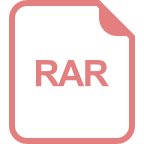
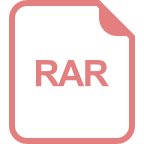
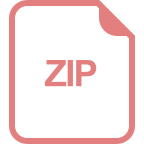
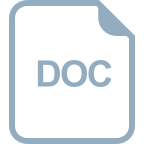
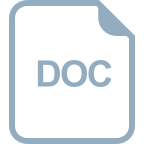
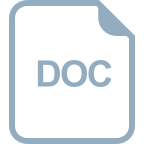
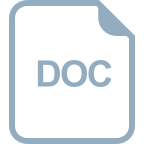
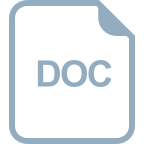
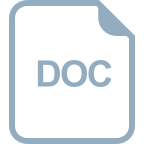
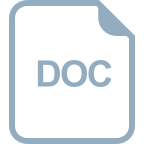
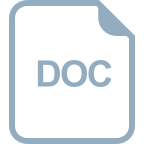
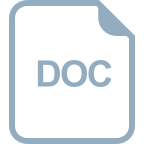
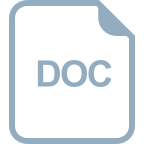
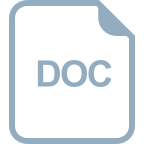
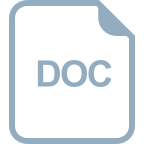
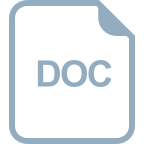
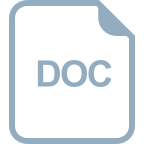