c++ openssl rsa加解密
时间: 2024-10-25 11:02:52 浏览: 13
在 C++ 中,使用 OpenSSL 库来进行 RSA 加密和解密操作,首先需要包含 OpenSSL 的头文件,并初始化库。以下是一个简单的示例,演示了如何创建 RSA 密钥对、加密数据以及解密数据:
```cpp
#include <openssl/pem.h>
#include <openssl/rsa.h>
// 创建 RSA 密钥对
RSA* generate_rsa_keypair() {
RSA* key = RSA_new();
BIGNUM* e = BN_new();
BN_set_word(e, RSA_F4);
RSA_generate_key_ex(key, 2048, e, NULL); // 生成 2048 比特的密钥
BN_free(e);
return key;
}
// 将 RSA 密钥转换成 PEM 格式字符串
std::string rsa_to_pem(RSA* key) {
BIO* bio = BIO_new(BIO_s_mem());
PEM_write_bio_RSAPrivateKey(bio, key, NULL, NULL);
char* pem_data = BIO_get_mem_ptr(bio);
size_t pem_len = BIO_get_mem_size(bio);
BIO_free_all(bio);
std::string pem(pem_data, pem_len);
OPENSSL_free(pem_data);
return pem;
}
// 加密数据
std::string encrypt(const std::string& plaintext, RSA* public_key) {
unsigned char ciphertext[1024];
int len = RSA_public_encrypt(strlen(plaintext.c_str()), (unsigned char*)plaintext.c_str(), ciphertext, public_key, RSA_PKCS1_OAEP_PADDING);
if (len <= 0)
throw std::runtime_error("Encryption failed");
return std::string((char*)ciphertext, len);
}
// 解密数据
stdstring decrypt(const std::string& ciphertext, RSA* private_key) {
unsigned char decrypted[1024];
int len = RSA_private_decrypt(strlen(ciphertext.c_str()), (unsigned char*)ciphertext.c_str(), decrypted, private_key, RSA_PKCS1_OAEP_PADDING);
if (len <= 0)
throw std::runtime_error("Decryption failed");
return std::string((char*)decrypted, len);
}
阅读全文
相关推荐
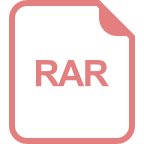
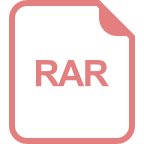
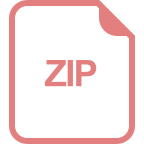




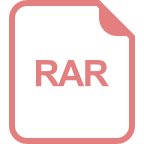
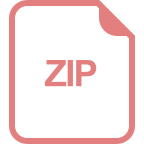







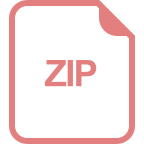
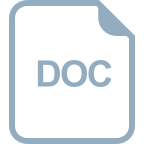
