java发post请求如何忽略证书
时间: 2023-07-18 11:50:42 浏览: 125
在 Java 中发送 POST 请求时,可以使用 HttpURLConnection 类或 HttpClient 类。不同的类库有不同的实现方式,以下是两种常见的忽略证书的方法:
1. 使用 HttpURLConnection 类
```
// 创建 SSLContext 对象,并指定信任所有证书
SSLContext sslContext = SSLContext.getInstance("SSL");
sslContext.init(null, new TrustManager[]{new X509TrustManager() {
public void checkClientTrusted(X509Certificate[] chain, String authType) throws CertificateException {
}
public void checkServerTrusted(X509Certificate[] chain, String authType) throws CertificateException {
}
public X509Certificate[] getAcceptedIssuers() {
return new X509Certificate[0];
}
}}, new SecureRandom());
// 设置默认的 SSLSocketFactory 和 HostnameVerifier
HttpsURLConnection.setDefaultSSLSocketFactory(sslContext.getSocketFactory());
HttpsURLConnection.setDefaultHostnameVerifier((hostname, session) -> true);
// 创建 URL 对象,并发送 POST 请求
URL url = new URL("https://example.com/api");
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("POST");
conn.setDoOutput(true);
// 添加请求参数
Map<String, String> params = new HashMap<>();
params.put("param1", "value1");
params.put("param2", "value2");
String requestBody = new Gson().toJson(params);
OutputStream os = conn.getOutputStream();
os.write(requestBody.getBytes());
os.flush();
os.close();
// 读取响应数据
BufferedReader br = new BufferedReader(new InputStreamReader(conn.getInputStream()));
StringBuilder sb = new StringBuilder();
String line;
while ((line = br.readLine()) != null) {
sb.append(line);
}
br.close();
String responseBody = sb.toString();
System.out.println(responseBody);
```
在上述代码中,我们通过创建一个 TrustManager 对象来信任所有证书。然后,我们设置默认的 SSLSocketFactory 和 HostnameVerifier,使其信任所有主机名和证书。这样就可以忽略证书验证,发送 POST 请求。
2. 使用 HttpClient 类
```
// 创建 SSLContext 对象,并指定信任所有证书
SSLContext sslContext = SSLContext.getInstance("SSL");
sslContext.init(null, new TrustManager[]{new X509TrustManager() {
public void checkClientTrusted(X509Certificate[] chain, String authType) throws CertificateException {
}
public void checkServerTrusted(X509Certificate[] chain, String authType) throws CertificateException {
}
public X509Certificate[] getAcceptedIssuers() {
return new X509Certificate[0];
}
}}, new SecureRandom());
// 创建 HttpClient 对象
CloseableHttpClient httpClient = HttpClients.custom()
.setSSLContext(sslContext)
.setSSLHostnameVerifier(NoopHostnameVerifier.INSTANCE)
.build();
// 创建 HttpPost 对象
HttpPost httpPost = new HttpPost("https://example.com/api");
// 添加请求参数
Map<String, String> params = new HashMap<>();
params.put("param1", "value1");
params.put("param2", "value2");
String requestBody = new Gson().toJson(params);
StringEntity entity = new StringEntity(requestBody, ContentType.APPLICATION_JSON);
httpPost.setEntity(entity);
// 发送 POST 请求
CloseableHttpResponse httpResponse = httpClient.execute(httpPost);
// 读取响应数据
HttpEntity responseEntity = httpResponse.getEntity();
String responseBody = EntityUtils.toString(responseEntity);
System.out.println(responseBody);
// 关闭 HttpClient 和 HttpResponse
httpResponse.close();
httpClient.close();
```
在上述代码中,我们通过创建一个 TrustManager 对象来信任所有证书。然后,我们设置 SSLContext 和 SSLHostnameVerifier,使其信任所有主机名和证书。这样就可以忽略证书验证,发送 POST 请求。
阅读全文
相关推荐
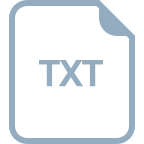
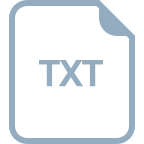
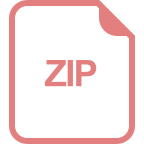



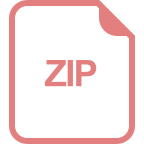
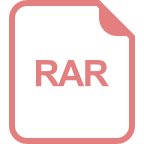
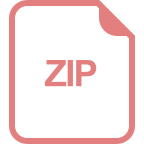
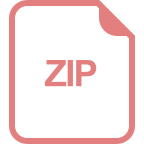
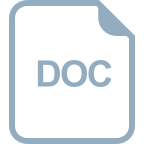
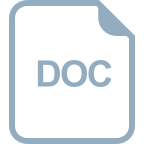
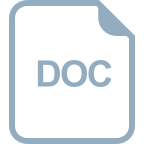
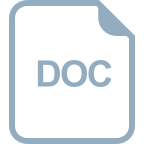


