许多应用程序,如字处理软件,邮件客户端等,都包含了单词检查特性。单词检查是根据字典,找出输入文本中拼错的单词,我们认为凡是不出现在字典中的单词都是错误单词。不仅如此,一些检查程序还能给出类似拼错单词的修改建议单词。 例如字典由下面几个单词组成: bake cake main rain vase 如果输入文件中有词vake ,检查程序就能发现其是一个错误的单词,并且给出 bake, cake或vase做为修改建议单词。 修改建议单词可以采用如下生成技术: (1)在每一个可能位置插入‘a-'z'中的一者 (2)删除单词中的一个字符 (3)用‘a'-'z'中的一者取代单词中的任一字符 很明显拼写检查程序的核心操作是在字典中查找某个单词,如果字典很大,性能无疑是非常关键的。 你写的程序要求读入字典文件,然后对一个输入文件的单词进行检查,列出其中的错误单词并给出修改建议。 本题要求使用使用二叉排序树维护字典。用c语言实现该问题
时间: 2024-03-15 22:47:13 浏览: 56
以下是使用二叉排序树实现拼写检查程序的C语言代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <ctype.h>
#define MAX_WORD_LEN 50
struct node {
char word[MAX_WORD_LEN];
struct node *left;
struct node *right;
};
struct node *create_node(char *word) {
struct node *new_node = (struct node*)malloc(sizeof(struct node));
strcpy(new_node->word, word);
new_node->left = NULL;
new_node->right = NULL;
return new_node;
}
struct node *insert(struct node *root, char *word) {
if (root == NULL) {
return create_node(word);
}
int cmp = strcmp(word, root->word);
if (cmp < 0) {
root->left = insert(root->left, word);
} else if (cmp > 0) {
root->right = insert(root->right, word);
}
return root;
}
void print_suggestions(struct node *root, char *word) {
if (root == NULL) {
return;
}
int cmp = strcmp(word, root->word);
if (cmp == 0) {
return;
} else if (cmp < 0) {
print_suggestions(root->left, word);
} else {
print_suggestions(root->right, word);
}
int len1 = strlen(word);
int len2 = strlen(root->word);
if (len1 == len2) {
int diff = 0;
for (int i = 0; i < len1; i++) {
if (word[i] != root->word[i]) {
diff++;
}
}
if (diff == 1) {
printf(" %s\n", root->word);
}
} else if (len1 == len2 + 1) {
int i = 0, j = 0;
while (i < len1 && j < len2) {
if (word[i] != root->word[j]) {
if (i != j) {
return;
}
i++;
} else {
i++;
j++;
}
}
printf(" %s\n", root->word);
} else if (len1 == len2 - 1) {
int i = 0, j = 0;
while (i < len1 && j < len2) {
if (word[i] != root->word[j]) {
if (i != j) {
return;
}
j++;
} else {
i++;
j++;
}
}
printf(" %s\n", root->word);
} else if (len1 == len2) {
int diff = 0;
for (int i = 0; i < len1; i++) {
if (word[i] != root->word[i]) {
diff++;
}
}
if (diff == 1) {
printf(" %s\n", root->word);
}
}
}
void check_word(struct node *root, char *word) {
struct node *cur = root;
while (cur != NULL) {
int cmp = strcmp(word, cur->word);
if (cmp == 0) {
return;
} else if (cmp < 0) {
cur = cur->left;
} else {
cur = cur->right;
}
}
printf("%s:\n", word);
printf(" Not found in dictionary.\n");
printf(" Suggestions:\n");
print_suggestions(root, word);
}
void read_dict(struct node **root, char *filename) {
FILE *fp = fopen(filename, "r");
if (fp == NULL) {
printf("Error opening file %s\n", filename);
exit(1);
}
char word[MAX_WORD_LEN];
while (fscanf(fp, "%s", word) != EOF) {
*root = insert(*root, word);
}
fclose(fp);
}
void check_file(struct node *root, char *filename) {
FILE *fp = fopen(filename, "r");
if (fp == NULL) {
printf("Error opening file %s\n", filename);
exit(1);
}
char word[MAX_WORD_LEN];
while (fscanf(fp, "%s", word) != EOF) {
for (int i = 0; i < strlen(word); i++) {
word[i] = tolower(word[i]);
}
check_word(root, word);
}
fclose(fp);
}
int main(int argc, char *argv[]) {
if (argc != 3) {
printf("Usage: %s dictionary_file input_file\n", argv[0]);
exit(1);
}
struct node *root = NULL;
read_dict(&root, argv[1]);
check_file(root, argv[2]);
return 0;
}
```
主要思路是先读入字典文件,构建二叉排序树,然后对输入文件中的每个单词进行拼写检查,如果该单词不在字典中,则输出其为错误单词,并列举出可能的修改建议单词。对于每个修改建议单词,使用一些简单的算法进行匹配,判断其是否和输入单词相似。
阅读全文
相关推荐
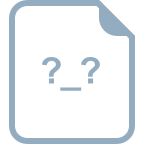
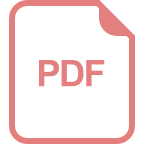

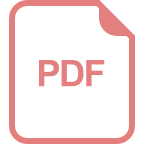
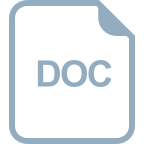
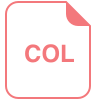
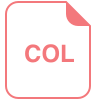
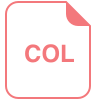
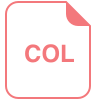
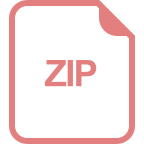
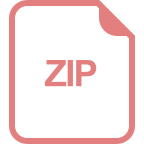
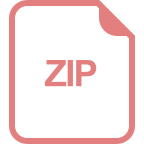
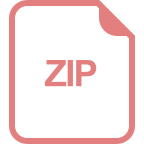
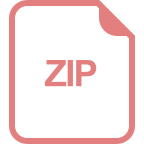
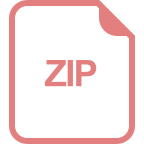
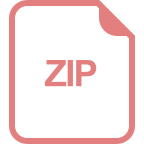
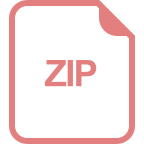