C➕➕编写一个程序实现顺序栈的各种基本运算
时间: 2023-09-30 17:06:07 浏览: 142
好的,下面是一个基本的顺序栈的实现,包括 push、pop、peek、isEmpty、isFull 等基本运算:
```c++
#include<iostream>
using namespace std;
#define MAX_SIZE 100 // 定义栈的最大容量
class SeqStack {
private:
int data[MAX_SIZE]; // 存储栈中数据的数组
int top; // 栈顶指针
public:
SeqStack(); // 构造函数
bool push(int x); // 入栈操作
int pop(); // 出栈操作
int peek(); // 获取栈顶元素
bool isEmpty(); // 判断栈是否为空
bool isFull(); // 判断栈是否已满
};
SeqStack::SeqStack()
{
top = -1;
}
bool SeqStack::push(int x)
{
if (isFull()) {
return false;
}
top++;
data[top] = x;
return true;
}
int SeqStack::pop()
{
if (isEmpty()) {
return -1;
}
int tmp = data[top];
top--;
return tmp;
}
int SeqStack::peek()
{
if (isEmpty()) {
return -1;
}
return data[top];
}
bool SeqStack::isEmpty()
{
return top == -1;
}
bool SeqStack::isFull()
{
return top == MAX_SIZE - 1;
}
int main()
{
SeqStack s;
s.push(1);
s.push(2);
s.push(3);
cout << s.pop() << endl; // 输出 3
cout << s.peek() << endl; // 输出 2
return 0;
}
```
上述代码中,我们定义了一个 `SeqStack` 类,包括私有成员变量 `data` 数组和 `top` 栈顶指针,以及公有成员函数 `push`、`pop`、`peek`、`isEmpty` 和 `isFull` 等基本运算。其中,`push` 和 `pop` 分别实现了入栈和出栈操作,`peek` 可以获取栈顶元素,`isEmpty` 和 `isFull` 判断栈是否为空或已满。在 `main` 函数中,我们使用 `SeqStack` 类创建一个栈对象 `s`,并进行了入栈、出栈和获取栈顶元素的操作,最终输出结果。
阅读全文
相关推荐
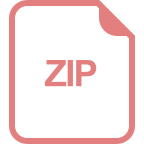















