python 使用库函数计算矩阵中每个数据的平方和
时间: 2023-07-15 18:15:50 浏览: 132
可以使用numpy库来计算矩阵中每个数据的平方和,示例如下:
```python
import numpy as np
# 定义一个 3*3 的矩阵
matrix = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
# 计算矩阵中每个数据的平方和
sum_of_squares = np.sum(np.square(matrix))
print(sum_of_squares) # 输出结果为 285
```
在上面的示例中,我们首先使用numpy库创建了一个3*3的矩阵,然后使用np.square()函数对矩阵中的每个元素进行平方操作,再使用np.sum()函数求和即可得到矩阵中每个数据的平方和。
相关问题
不使用python库函数计算图像均方差
计算图像的均方差(Mean Squared Error, MSE)通常涉及到像素值的统计处理,如果你不想使用Python的库函数,可以手动编写一个简单的循环来完成这个过程。以下是一个基本步骤:
1. **读取图像**:首先,你需要将图像加载到一个二维数组中,每个元素代表一个像素的灰度值。
```python
# 假设img是你的图像数据,通常是numpy数组
image = img.astype('float')
```
2. **计算平均值**:对所有像素求平均,得到均值(mean)。
```python
image_mean = image.mean()
```
3. **计算差平方**:对于每个像素,减去平均值,然后平方,得到每个像素的偏差平方。
```python
squared_differences = (image - image_mean) ** 2
```
4. **计算均方差**:最后,计算所有偏差平方的平均值即得MSE。
```python
mse = squared_differences.mean()
```
完整代码示例:
```python
import numpy as np
def calculate_mse(image):
if not isinstance(image, np.ndarray):
raise ValueError("Input should be a numpy array.")
# ...上述步骤...
mse_value = calculate_mse(image)
```
python计算误差平方和代码库函数
在Python中,可以使用numpy库中的`sum()`函数来计算误差平方和。具体步骤如下:
1. 导入numpy库
```python
import numpy as np
```
2. 定义实际值和预测值
```python
y_true = np.array([1, 2, 3, 4, 5])
y_pred = np.array([1.2, 2.3, 2.8, 4.1, 4.9])
```
3. 计算误差
```python
error = y_true - y_pred
```
4. 计算误差平方和
```python
mse = np.sum(error ** 2)
```
完整代码如下:
```python
import numpy as np
# 定义实际值和预测值
y_true = np.array([1, 2, 3, 4, 5])
y_pred = np.array([1.2, 2.3, 2.8, 4.1, 4.9])
# 计算误差
error = y_true - y_pred
# 计算误差平方和
mse = np.sum(error ** 2)
print("误差平方和为:", mse)
```
阅读全文
相关推荐
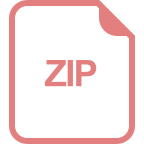
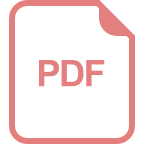
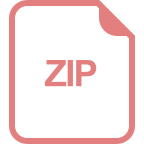













