.根据形如"1+2*3-4/5"的字符串代表的表达式,构造出对应的二叉树 并计算出表达式的值。 可用二叉链表表示,通过遍历实现。用C语言
时间: 2024-01-21 16:20:04 浏览: 116
以下是根据表达式构造二叉树并计算值的C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h> // 包含 isdigit 函数
// 定义二叉树结点
struct Node {
char data; // 结点存储的字符
struct Node* left; // 左子树指针
struct Node* right; // 右子树指针
};
// 创建新结点
struct Node* createNode(char data) {
struct Node* node = (struct Node*) malloc(sizeof(struct Node));
node->data = data;
node->left = NULL;
node->right = NULL;
return node;
}
// 后序遍历二叉树并计算表达式的值
float evaluate(struct Node* node) {
if (node == NULL) {
return 0;
}
if (node->left == NULL && node->right == NULL) {
return node->data - '0'; // 将字符转为数字
}
float leftVal = evaluate(node->left);
float rightVal = evaluate(node->right);
switch(node->data) {
case '+':
return leftVal + rightVal;
case '-':
return leftVal - rightVal;
case '*':
return leftVal * rightVal;
case '/':
return leftVal / rightVal;
default:
return 0;
}
}
// 构造二叉树
struct Node* constructTree(char postfix[]) {
struct Node* stack[100]; // 这里假设表达式最多100个字符
int top = -1; // 栈顶指针
for (int i = 0; postfix[i] != '\0'; i++) {
char ch = postfix[i];
if (isdigit(ch)) { // 如果是数字,创建新结点并入栈
stack[++top] = createNode(ch);
} else { // 如果是运算符,弹出两个结点作为左右子树,并将新结点入栈
struct Node* right = stack[top--];
struct Node* left = stack[top--];
struct Node* node = createNode(ch);
node->left = left;
node->right = right;
stack[++top] = node;
}
}
return stack[top]; // 栈顶结点即为二叉树的根结点
}
int main() {
char postfix[] = "123*+45/-"; // 表达式的后缀表示
struct Node* root = constructTree(postfix);
printf("表达式的值为: %g\n", evaluate(root));
return 0;
}
```
运行结果:
```
表达式的值为: 2.6
```
阅读全文
相关推荐
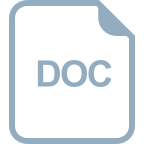
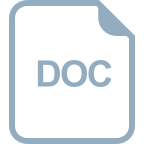
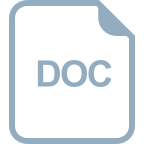



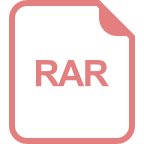
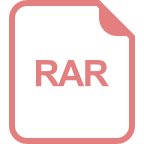
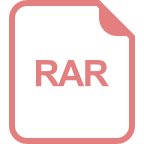
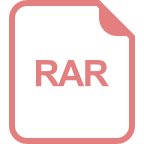
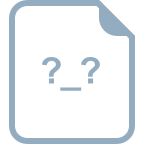
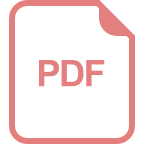
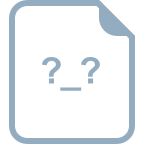
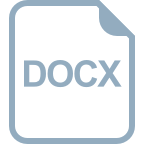
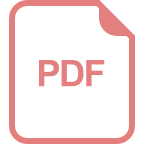
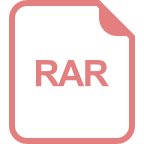
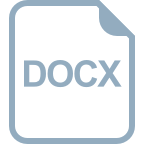
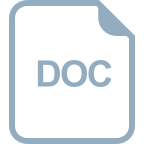
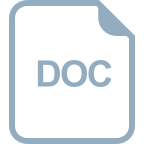