随机产生80个整数构成的递增序列,使用折半查找算法查找指定的整数,并统计比较次数。提示:可用 a[i] = a[i-1] + rand() % 10 + 1产生递增序列
时间: 2024-01-22 10:21:09 浏览: 50
好的,我可以给你提供一个简单的 Python 代码来实现这个功能。
```python
import random
# 生成递增序列
a = [random.randint(1, 10)]
for i in range(1, 80):
a.append(a[i-1] + random.randint(1, 10))
# 折半查找算法
def binary_search(arr, target):
low = 0
high = len(arr) - 1
count = 0
while low <= high:
mid = (low + high) // 2
count += 1
if arr[mid] == target:
return mid, count
elif arr[mid] < target:
low = mid + 1
else:
high = mid - 1
return -1, count
# 查找指定整数
target = random.choice(a)
index, count = binary_search(a, target)
# 输出结果
if index != -1:
print(f"在序列中找到了 {target},下标为 {index},比较次数为 {count}")
else:
print(f"在序列中未找到 {target},比较次数为 {count}")
```
这个代码首先通过 `random.randint(1, 10)` 生成随机的递增序列,长度为 80。然后使用 `binary_search` 函数实现折半查找算法,在序列中查找指定整数 `target`。最后输出查找结果和比较次数。
注意,由于是随机生成序列和目标整数,每次运行结果可能不同。
阅读全文
相关推荐










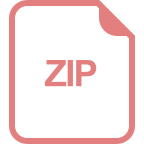
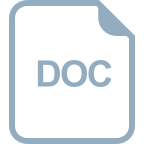
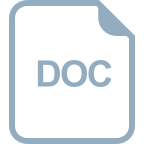