随机产生1000个整数构成的递增序列,使用折半查找算法查找指定的整数,并统计比较次数。提示:可用 a[i] = a[i-1] + rand() % 10 + 1产生递增序列。(使用c语言)
时间: 2024-02-09 16:13:17 浏览: 23
好的,我来回答你的问题。
首先,我们需要了解折半查找算法的基本思想。折半查找算法也叫二分查找算法,是一种利用有序数组的特性进行查找的算法,其基本思想是:每次查找时,将待查找的区间的中间元素与目标元素进行比较,如果相等则查找成功;如果目标元素小于中间元素,则在左半区间中继续查找;如果目标元素大于中间元素,则在右半区间中继续查找。每次查找都可以将待查找的区间缩小一半,因此时间复杂度为 O(logn)。
接下来,我们可以使用以下代码来生成随机的递增序列:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define SIZE 1000
int main() {
int a[SIZE];
int i;
srand(time(NULL));
a[0] = rand() % 100;
for (i = 1; i < SIZE; i++) {
a[i] = a[i - 1] + rand() % 10 + 1;
}
// 打印生成的递增序列
for (i = 0; i < SIZE; i++) {
printf("%d ", a[i]);
}
printf("\n");
return 0;
}
```
接下来,我们可以使用以下代码来实现折半查找算法,并统计比较次数:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define SIZE 1000
int binary_search(int a[], int size, int key, int *cmp_count) {
int left = 0, right = size - 1, mid;
while (left <= right) {
mid = (left + right) / 2;
(*cmp_count)++;
if (a[mid] == key) {
return mid;
} else if (a[mid] > key) {
right = mid - 1;
} else {
left = mid + 1;
}
}
return -1;
}
int main() {
int a[SIZE];
int i, key, index, cmp_count = 0;
srand(time(NULL));
a[0] = rand() % 100;
for (i = 1; i < SIZE; i++) {
a[i] = a[i - 1] + rand() % 10 + 1;
}
// 打印生成的递增序列
for (i = 0; i < SIZE; i++) {
printf("%d ", a[i]);
}
printf("\n");
// 生成随机的查找数字
key = rand() % 1000;
// 使用折半查找算法查找数字并统计比较次数
index = binary_search(a, SIZE, key, &cmp_count);
if (index == -1) {
printf("The number %d was not found in the array.\n", key);
} else {
printf("The number %d was found at index %d in the array.\n", key, index);
}
printf("The number of comparisons used in the search is %d.\n", cmp_count);
return 0;
}
```
这样,我们就完成了随机产生1000个整数构成的递增序列,并使用折半查找算法查找指定的整数,并统计比较次数的操作。
相关推荐
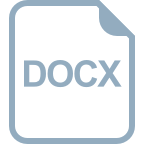





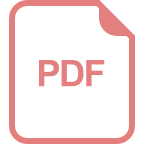
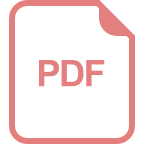
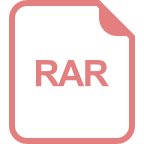
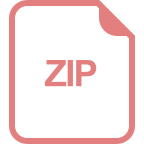
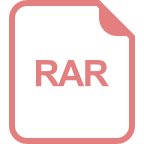