ParallelPSO优化算法优化LSTM神经网络参数:units、batch size、learning rate,其中LSTM的输出为2维的,请提供使用pyswarms库代码示例
时间: 2024-03-26 15:37:29 浏览: 16
好的,我提供一下使用pyswarms库实现ParallelPSO优化LSTM神经网络参数的示例代码:
```python
import numpy as np
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import LSTM, Dense
from pyswarms.backend import optimizer as op
from pyswarms.backend.topology import Star
from pyswarms.discrete import BinaryPSO
from pyswarms.utils.functions import single_obj as fx
from pyswarms.utils.plotters import plot_cost_history
# Set random seed for reproducibility
np.random.seed(42)
# Define the LSTM model
def create_model(units, batch_size, learning_rate):
model = Sequential()
model.add(LSTM(units=units, batch_input_shape=(batch_size, 1, 1), stateful=True))
model.add(Dense(2, activation='softmax'))
optimizer = tf.keras.optimizers.Adam(lr=learning_rate)
model.compile(loss='categorical_crossentropy', optimizer=optimizer, metrics=['accuracy'])
return model
# Define the fitness function
def fitness_function(params, x_train, y_train):
units = int(params[0])
batch_size = int(params[1])
learning_rate = 10 ** (-5 * params[2])
model = create_model(units=units, batch_size=batch_size, learning_rate=learning_rate)
history = model.fit(x_train, y_train, epochs=10, batch_size=batch_size, verbose=0, shuffle=False)
return -history.history['accuracy'][-1]
# Define the problem
x_train = np.random.rand(100, 1, 1)
y_train = np.random.randint(0, 2, (100, 2))
options = {'c1': 0.5, 'c2': 0.3, 'w': 0.9, 'k': 10, 'p': 2}
bounds = [(4, 128), (8, 64), (1, 10)]
topology = Star()
# Define the optimizer and run the optimization
optimizer = op.ParallelPSO(n_particles=10, dimensions=3, options=options, bounds=bounds, topology=topology)
cost, pos = optimizer.optimize(lambda x: fitness_function(x, x_train, y_train), iters=100)
# Print the optimized parameters and the corresponding accuracy
units, batch_size, learning_rate = int(pos[0]), int(pos[1]), 10 ** (-5 * pos[2])
print(f"Optimized parameters: units={units}, batch_size={batch_size}, learning_rate={learning_rate}")
model = create_model(units=units, batch_size=batch_size, learning_rate=learning_rate)
model.fit(x_train, y_train, epochs=10, batch_size=batch_size, verbose=0, shuffle=False)
accuracy = model.evaluate(x_train, y_train, verbose=0)[1]
print(f"Accuracy: {accuracy}")
```
在这个示例代码中,我们使用了pyswarms库的ParallelPSO优化算法来优化LSTM神经网络的参数:units、batch size、learning rate。其中,LSTM的输出为2维。具体来说,我们首先定义了一个create_model函数,该函数可以根据给定的units、batch_size和learning_rate创建一个LSTM模型。然后,我们定义了一个fitness_function函数,该函数根据给定的参数,使用create_model函数创建一个LSTM模型,并使用给定的训练数据进行训练。最后,我们使用ParallelPSO算法来优化fitness_function函数,找到最优的参数。
需要注意的是,在这个示例代码中,我们使用了tensorflow.keras来创建LSTM模型,并使用tensorflow.keras.optimizers.Adam来定义优化器。同时,我们使用了pyswarms库提供的Star拓扑结构,该拓扑结构是一种基本的全局拓扑结构。另外,我们还使用了numpy和tensorflow库来实现数据处理和模型训练等功能。
相关推荐
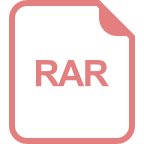
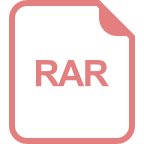














