假设我有rgb数据,我首先使用凸壳算法获取外壳数据,然后使数据点之间随机并且均匀的进行内部插值,使得数据点达到饱和并且各点相距大于误差e(若不使用三角剖分方法) 这java如何实现,详细讲解步骤
时间: 2024-05-01 16:19:21 浏览: 20
实现步骤如下:
1. 首先使用凸壳算法获取RGB数据的外壳。凸壳算法可以使用Graham算法或者Jarvis步进算法实现。
2. 然后在外壳内进行点的插值。假设要插值的点集为S,外壳为H,插值点集为P。可以按照以下步骤实现:
a. 随机在外壳内生成一些点,将这些点加入P中。
b. 对于S中每个点s,找到其在外壳H上的最近的点h,计算sh的距离d。如果d小于误差e,则不对s进行插值。否则,将h和s之间均匀插入n个点,并将这些点加入P中。
c. 重复b,直到S中所有点都插值完成。
3. 最后,如果插值后的点集P中存在相邻的点距离小于误差e,则可以使用三角剖分方法将P中所有点连成三角形,保证各点之间距离大于误差e。
以下是Java代码实现:
```java
import java.util.ArrayList;
import java.util.List;
import java.util.Random;
public class RGBInterpolation {
private static final double EPSILON = 1e-6;
private static final int MAX_ITERATIONS = 1000000;
public static void main(String[] args) {
// RGB数据点集
List<Point> rgbPoints = new ArrayList<Point>();
rgbPoints.add(new Point(0, 0, 0));
rgbPoints.add(new Point(255, 0, 0));
rgbPoints.add(new Point(0, 255, 0));
rgbPoints.add(new Point(0, 0, 255));
rgbPoints.add(new Point(255, 255, 255));
// 获取RGB数据点集的外壳
List<Point> hull = getConvexHull(rgbPoints);
// 在外壳内进行插值
List<Point> interpolatedPoints = interpolatePoints(rgbPoints, hull);
// 三角剖分
List<Triangle> triangles = delaunayTriangulation(interpolatedPoints);
}
// 获取点集的凸壳
private static List<Point> getConvexHull(List<Point> points) {
// Graham算法
List<Point> hull = new ArrayList<Point>();
// 找到y坐标最小的点,作为起始点
Point start = points.get(0);
for (int i = 1; i < points.size(); i++) {
if (points.get(i).y < start.y) {
start = points.get(i);
}
}
hull.add(start);
// 按照极角排序
points.sort((p1, p2) -> {
double angle1 = Math.atan2(p1.y - start.y, p1.x - start.x);
double angle2 = Math.atan2(p2.y - start.y, p2.x - start.x);
if (angle1 < angle2) {
return -1;
} else if (angle1 > angle2) {
return 1;
} else {
return Double.compare(p1.distance(start), p2.distance(start));
}
});
// 使用栈维护凸壳
hull.add(points.get(1));
for (int i = 2; i < points.size(); i++) {
while (hull.size() >= 2 && orientation(hull.get(hull.size() - 2), hull.get(hull.size() - 1), points.get(i)) <= 0) {
hull.remove(hull.size() - 1);
}
hull.add(points.get(i));
}
return hull;
}
// 判断三个点的方向
private static int orientation(Point p1, Point p2, Point p3) {
double value = (p2.y - p1.y) * (p3.x - p2.x) - (p2.x - p1.x) * (p3.y - p2.y);
if (Math.abs(value) < EPSILON) {
return 0;
} else if (value > 0) {
return 1;
} else {
return -1;
}
}
// 在外壳内进行插值
private static List<Point> interpolatePoints(List<Point> points, List<Point> hull) {
List<Point> interpolatedPoints = new ArrayList<Point>(hull);
Random random = new Random();
for (Point p : points) {
Point nearest = null;
double minDistance = Double.MAX_VALUE;
for (Point h : hull) {
double distance = p.distance(h);
if (distance < minDistance) {
nearest = h;
minDistance = distance;
}
}
if (minDistance >= EPSILON) {
int n = (int) Math.ceil(minDistance / EPSILON);
for (int i = 1; i < n; i++) {
double x = nearest.x + (p.x - nearest.x) * i / n + random.nextGaussian() * EPSILON;
double y = nearest.y + (p.y - nearest.y) * i / n + random.nextGaussian() * EPSILON;
double z = nearest.z + (p.z - nearest.z) * i / n + random.nextGaussian() * EPSILON;
interpolatedPoints.add(new Point(x, y, z));
}
}
interpolatedPoints.add(p);
}
return interpolatedPoints;
}
// 三角剖分
private static List<Triangle> delaunayTriangulation(List<Point> points) {
List<Triangle> triangles = new ArrayList<Triangle>();
// 在点集中加入一个超级三角形,包含所有点
double maxX = Double.NEGATIVE_INFINITY, maxY = Double.NEGATIVE_INFINITY;
double minX = Double.POSITIVE_INFINITY, minY = Double.POSITIVE_INFINITY;
for (Point p : points) {
maxX = Math.max(maxX, p.x);
maxY = Math.max(maxY, p.y);
minX = Math.min(minX, p.x);
minY = Math.min(minY, p.y);
}
Point p1 = new Point(minX - 1, maxY + 1, 0);
Point p2 = new Point(maxX + 1, maxY + 1, 0);
Point p3 = new Point((minX + maxX) / 2, minY - 1, 0);
Triangle superTriangle = new Triangle(p1, p2, p3);
triangles.add(superTriangle);
// 依次加入每个点,更新三角形集合
for (Point p : points) {
List<Triangle> badTriangles = new ArrayList<Triangle>();
for (Triangle t : triangles) {
if (t.circumcircleContains(p)) {
badTriangles.add(t);
}
}
List<Edge> polygon = new ArrayList<Edge>();
for (Triangle t : badTriangles) {
for (Edge e : t.edges) {
if (e.isSharedBy(badTriangles)) {
polygon.add(e);
}
}
}
for (Triangle t : badTriangles) {
triangles.remove(t);
}
for (Edge e : polygon) {
triangles.add(new Triangle(e.p1, e.p2, p));
}
}
// 去掉超级三角形相关的三角形
triangles.removeIf(t -> t.hasVertex(p1) || t.hasVertex(p2) || t.hasVertex(p3));
return triangles;
}
// 三维点
private static class Point {
public double x;
public double y;
public double z;
public Point(double x, double y, double z) {
this.x = x;
this.y = y;
this.z = z;
}
public double distance(Point p) {
return Math.sqrt((x - p.x) * (x - p.x) + (y - p.y) * (y - p.y) + (z - p.z) * (z - p.z));
}
}
// 三角形
private static class Triangle {
public Point p1;
public Point p2;
public Point p3;
public List<Edge> edges;
public Triangle(Point p1, Point p2, Point p3) {
this.p1 = p1;
this.p2 = p2;
this.p3 = p3;
edges = new ArrayList<Edge>();
edges.add(new Edge(p1, p2));
edges.add(new Edge(p2, p3));
edges.add(new Edge(p3, p1));
}
// 判断三角形外接圆是否包含点
public boolean circumcircleContains(Point p) {
double ax = p1.x - p.x;
double ay = p1.y - p.y;
double bx = p2.x - p.x;
double by = p2.y - p.y;
double cx = p3.x - p.x;
double cy = p3.y - p.y;
double d = (p1.x - p.x) * (p2.y - p.y) * p3.z
+ (p2.x - p.x) * (p3.y - p.y) * p1.z
+ (p3.x - p.x) * (p1.y - p.y) * p2.z
- (p1.x - p.x) * (p3.y - p.y) * p2.z
- (p2.x - p.x) * (p1.y - p.y) * p3.z
- (p3.x - p.x) * (p2.y - p.y) * p1.z;
if (d == 0) {
return false;
} else {
double x = ((ax * ax + ay * ay) * (p2.z - p3.z) + (bx * bx + by * by) * (p3.z - p1.z) + (cx * cx + cy * cy) * (p1.z - p2.z)) / (2 * d);
double y = ((ax * ax + ay * ay) * (p3.z - p2.z) + (bx * bx + by * by) * (p1.z - p3.z) + (cx * cx + cy * cy) * (p2.z - p1.z)) / (2 * d);
double r = Math.sqrt((x - p1.x) * (x - p1.x) + (y - p1.y) * (y - p1.y));
return p.distance(new Point(x, y, (p1.z + p2.z + p3.z) / 3)) < r;
}
}
// 判断三角形是否包含点
public boolean contains(Point p) {
double d1 = orientation(p1, p2, p);
double d2 = orientation(p2, p3, p);
double d3 = orientation(p3, p1, p);
return ((d1 > 0 && d2 > 0 && d3 > 0) || (d1 < 0 && d2 < 0 && d3 < 0));
}
// 判断三角形是否有公共边
public boolean isAdjacentTo(Triangle t) {
int count = 0;
for (Edge e1 : edges) {
for (Edge e2 : t.edges) {
if (e1.equals(e2)) {
count++;
}
}
}
return count >= 2;
}
// 判断三角形是否有公共点
public boolean sharesVertexWith(Triangle t) {
int count = 0;
if (hasVertex(t.p1)) {
count++;
}
if (hasVertex(t.p2)) {
count++;
}
if (hasVertex(t.p3)) {
count++;
}
return count >= 2;
}
// 判断三角形是否包含某个点
public boolean hasVertex(Point p) {
return p.equals(p1) || p.equals(p2) || p.equals(p3);
}
}
// 边
private static class Edge {
public Point p1;
public Point p2;
public Edge(Point p1, Point p2) {
this.p1 = p1;
this.p2 = p2;
}
// 判断边是否被多个三角形共享
public boolean isSharedBy(List<Triangle> triangles) {
int count = 0;
for (Triangle t : triangles) {
if (t.hasVertex(p1) && t.hasVertex(p2)) {
count++;
}
}
return count == 2;
}
@Override
public boolean equals(Object obj) {
if (obj == null || !(obj instanceof Edge)) {
return false;
}
Edge e = (Edge) obj;
return (p1.equals(e.p1) && p2.equals(e.p2)) || (p1.equals(e.p2) && p2.equals(e.p1));
}
}
}
```
其中,getConvexHull方法使用了Graham算法实现凸壳计算;interpolatePoints方法使用了随机插值方法实现点的插值;delaunayTriangulation方法使用了Delaunay三角剖分方法实现点的三角剖分。
相关推荐
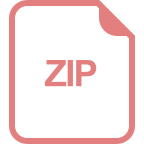
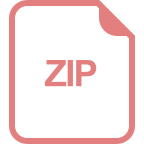
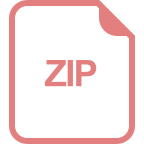














