用vs2015写一个.aspx的文件模拟购物车功能:2个商品购买页面(可互相切换),一个购物车(显示购物列表)。其中商品购买页面包含商品的名称、单价和购买数量,购物车能统计商品的单价、购买数量和总价,给出详细的书写过程代码
时间: 2024-01-21 14:17:50 浏览: 124
首先,我们需要创建一个ASP.NET Web应用程序,并添加一个.aspx页面和一个.aspx.cs文件。
在.aspx页面中,我们可以使用HTML和ASP.NET控件来创建购物车页面。以下是一个简单的模板:
```html
<!DOCTYPE html>
<html>
<head>
<title>购物车</title>
</head>
<body>
<h1>商品1</h1>
<p>单价:10元</p>
<form method="post">
<label>购买数量:</label>
<input type="number" name="product1_qty" value="0" min="0" step="1" /><br />
<input type="submit" name="add_to_cart" value="加入购物车" />
</form>
<h1>商品2</h1>
<p>单价:20元</p>
<form method="post">
<label>购买数量:</label>
<input type="number" name="product2_qty" value="0" min="0" step="1" /><br />
<input type="submit" name="add_to_cart" value="加入购物车" />
</form>
<h1>购物车</h1>
<table>
<thead>
<tr>
<th>商品名称</th>
<th>单价</th>
<th>数量</th>
<th>总价</th>
</tr>
</thead>
<tbody>
<%--购物车内容将在代码后面生成--%>
</tbody>
</table>
</body>
</html>
```
在.aspx.cs文件中,我们需要编写逻辑来处理购物车页面的交互。以下是一个简单的示例:
```csharp
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
public partial class ShoppingCart : System.Web.UI.Page
{
private Dictionary<string, int> cart = new Dictionary<string, int>();
protected void Page_Load(object sender, EventArgs e)
{
// 尝试从Session中获取购物车内容
if (Session["cart"] != null)
{
cart = (Dictionary<string, int>)Session["cart"];
}
// 处理用户提交的加入购物车请求
if (Request.Form["add_to_cart"] != null)
{
if (Request.Form["add_to_cart"] == "加入购物车")
{
// 处理商品1
if (Request.Form["product1_qty"] != null)
{
int qty = int.Parse(Request.Form["product1_qty"]);
if (qty > 0)
{
if (cart.ContainsKey("商品1"))
{
cart["商品1"] += qty;
}
else
{
cart["商品1"] = qty;
}
}
}
// 处理商品2
if (Request.Form["product2_qty"] != null)
{
int qty = int.Parse(Request.Form["product2_qty"]);
if (qty > 0)
{
if (cart.ContainsKey("商品2"))
{
cart["商品2"] += qty;
}
else
{
cart["商品2"] = qty;
}
}
}
// 将购物车内容保存到Session中
Session["cart"] = cart;
}
// 重新加载页面
Response.Redirect(Request.RawUrl);
}
// 生成购物车内容
foreach (var item in cart)
{
TableRow row = new TableRow();
TableCell nameCell = new TableCell();
nameCell.Text = item.Key;
row.Cells.Add(nameCell);
TableCell priceCell = new TableCell();
priceCell.Text = GetPrice(item.Key).ToString();
row.Cells.Add(priceCell);
TableCell qtyCell = new TableCell();
qtyCell.Text = item.Value.ToString();
row.Cells.Add(qtyCell);
TableCell totalCell = new TableCell();
totalCell.Text = (GetPrice(item.Key) * item.Value).ToString();
row.Cells.Add(totalCell);
cartTable.Rows.Add(row);
}
}
// 获取商品价格的方法
private decimal GetPrice(string productName)
{
if (productName == "商品1")
{
return 10m;
}
else if (productName == "商品2")
{
return 20m;
}
else
{
return 0m;
}
}
}
```
在这个示例中,我们创建了一个名为`cart`的字典来保存购物车的内容。在Page_Load事件中,我们首先尝试从Session中获取购物车内容,然后处理用户提交的加入购物车请求。如果用户单击“加入购物车”按钮,则我们将商品数量添加到购物车字典中,并将其保存到Session中。最后,我们使用`foreach`循环遍历购物车字典,并在表格中显示购物车内容。
请注意,此示例仅提供了一个简单的模板,并且没有进行严格的输入验证或异常处理。在实际的应用程序中,您需要根据需要添加更多的逻辑来确保数据的完整性和安全性。
阅读全文
相关推荐
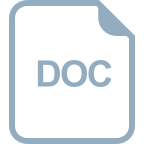
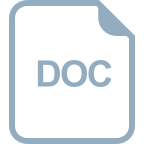
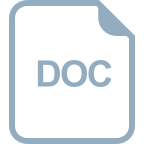
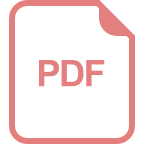
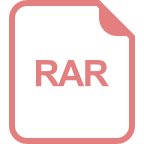
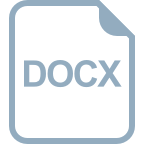
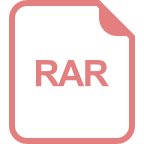
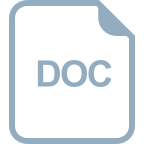
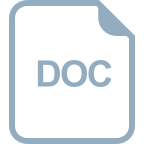
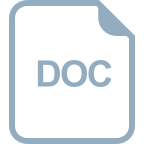


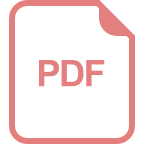
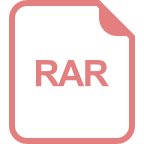
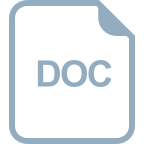
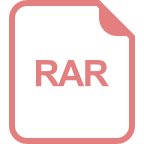
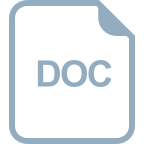
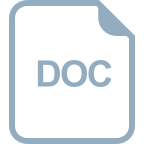
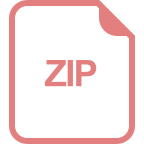