#include<stdio.h> typedef int Elemtype ; typedef struct LNode{ Elemtype data ; struct LNode *next ;}LNode,LinkList ; void CreatList_H(LinkList &L, int n){ L=new LNode ; LinkList P ; L->data=NULL ; L->next=NULL ; int i ; for(i=1;i<=n;i++){ P=new LNode ; scanf("%d",&P->data) ; P->next=L->next ; L->next=P ; } } void ReverseList(LinkList &L,int n){ LinkList P,R ; P=L->next->next ; R=L->next ; int i ; for(i=1;i<n;i++){ R->next=P->next ; P->next=L->next ; L->next=P->next ; P=R->next ; } } void PrintList(LinkList L, int n) { int i ; for(i=1;i<=n;i++){ L=L->next ; printf("%d\n",L->data) ; } } int main(){ LinkList L; int n ; scanf("%d",&n); if(n==0) { printf("list is empty") ; break ; } void CreatList( L, n) ; void ReverseList( L, n); void PrintList( L, n) ; return 0 ; }查错
时间: 2024-01-15 16:05:38 浏览: 74
这段代码存在以下问题:
1. 在主函数中,调用函数的语法错误,应该直接写函数名,即应该改为 `CreatList_H(L, n); ReverseList(L, n); PrintList(L, n);`
2. 在 `CreatList_H` 函数中,头节点的数据域应该是一个指针类型,而不是 `NULL`,即应该改为 `L->data = new LNode;`。
3. 在 `ReverseList` 函数中,对于第一个节点和第二个节点的指针赋值错误,应该是 `P = L->next->next; R = L->next;`,而不是 `P = L->next; R = L->next->next;`。
4. 在输出链表时,应该先判断链表是否为空,否则会出现空指针错误。可以在主函数中添加一个判断,即 `if(L == NULL) { printf("list is empty"); return 0; }`。
修改后的代码如下:
相关问题
#include<stdio.h> #include<math.h> #include<malloc.h> #define OK 1 #define FALSE 0 typedef int Status; typedef int Elemtype; typedef struct LNode { Elemtype data; struct LNode* next; }LNode,*LinkList; LinkList L; Status InitList(LinkList* L) { L = (LinkList)malloc(sizeof(LNode)); L->next = NULL; return OK; }
这段代码定义了一个单链表结构体,包含元素类型为`Elemtype`的数据和一个指向下一个结点的指针。同时定义了一个`LinkList`类型为结构体指针类型,以及一个`LNode`类型为单链表结点类型。
接下来定义了一个`L`指针作为链表的头结点,并实现了一个`InitList`函数用于初始化链表。在函数内,通过调用`malloc`函数为链表头结点分配内存空间,并将`next`成员初始化为`NULL`,表示当前链表为空。
需要注意的是,头文件的引用应该放在代码文件的最开始,而不是在函数定义之间。同时,宏定义中的`FALSE`没有被使用,建议将其删除或者使用。
请对以下代码进行修改#include<stdio.h> #include<math.h> #include<malloc.h> #include<stddef.h> #define OK 1 #define FALSE 0 typedef int Status; typedef int Elemtype; typedef struct LNode { Elemtype data; struct LNode* next; }LNode,*LinkList; LinkList L; Status InitList(LinkList*L) { L = (LinkList)malloc(sizeof(LNode)); L->next = NULL; return OK; }
这段代码的问题在于,`LinkList L`在全局作用域中定义,而在`InitList()`函数中又重新定义了一个`LinkList`类型的指针`L`,这会导致程序出现错误。应该将`LinkList L`的定义移动到`main()`函数中,并将其作为参数传递给`InitList()`函数。同时,函数返回值应该是`LinkList`类型的指针。
修改后的代码如下:
```
#include <stdio.h>
#include <math.h>
#include <malloc.h>
#include <stddef.h>
#define OK 1
#define FALSE 0
typedef int Status;
typedef int Elemtype;
typedef struct LNode {
Elemtype data;
struct LNode* next;
} LNode, *LinkList;
Status InitList(LinkList* L) {
*L = (LinkList)malloc(sizeof(LNode));
(*L)->next = NULL;
return OK;
}
int main() {
LinkList L;
InitList(&L);
return 0;
}
```
这里将`LinkList L`的定义移动到了`main()`函数中,并将其传递给`InitList()`函数。同时,函数返回值改为指向`LinkList`类型的指针。在`main()`函数中,我们调用`InitList()`函数来初始化链表,然后程序正常结束。
阅读全文
相关推荐
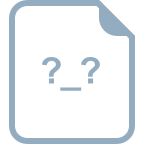
















