用c语言编写一个能执行集合的并、交和差运算的程序,集合的元素限定为小写字母字符
时间: 2023-06-13 08:03:44 浏览: 80
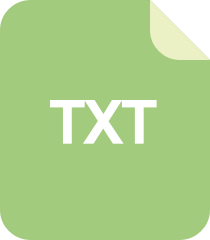
集合的并交补差
以下是一个用C语言编写的集合运算程序,可以进行并、交和差运算。集合的元素被限定为小写字母字符。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_SIZE 26 // 集合大小
void initializeSet(int set[MAX_SIZE]); // 初始化集合
void printSet(int set[MAX_SIZE]); // 打印集合
void unionSet(int set1[MAX_SIZE], int set2[MAX_SIZE], int result[MAX_SIZE]); // 集合并运算
void intersectSet(int set1[MAX_SIZE], int set2[MAX_SIZE], int result[MAX_SIZE]); // 集合交运算
void diffSet(int set1[MAX_SIZE], int set2[MAX_SIZE], int result[MAX_SIZE]); // 集合差运算
int main() {
int set1[MAX_SIZE], set2[MAX_SIZE], result[MAX_SIZE];
initializeSet(set1);
initializeSet(set2);
printf("请输入第一个集合(小写字母):\n");
char input1[MAX_SIZE];
scanf("%s", input1);
for (int i = 0; i < strlen(input1); i++) {
set1[input1[i] - 'a'] = 1;
}
printf("请输入第二个集合(小写字母):\n");
char input2[MAX_SIZE];
scanf("%s", input2);
for (int i = 0; i < strlen(input2); i++) {
set2[input2[i] - 'a'] = 1;
}
printf("第一个集合:");
printSet(set1);
printf("第二个集合:");
printSet(set2);
unionSet(set1, set2, result);
printf("并集:");
printSet(result);
intersectSet(set1, set2, result);
printf("交集:");
printSet(result);
diffSet(set1, set2, result);
printf("差集(第一个集合-第二个集合):");
printSet(result);
diffSet(set2, set1, result);
printf("差集(第二个集合-第一个集合):");
printSet(result);
return 0;
}
void initializeSet(int set[MAX_SIZE]) {
for (int i = 0; i < MAX_SIZE; i++) {
set[i] = 0;
}
}
void printSet(int set[MAX_SIZE]) {
printf("{");
for (int i = 0; i < MAX_SIZE; i++) {
if (set[i]) {
printf("%c", i + 'a');
}
}
printf("}\n");
}
void unionSet(int set1[MAX_SIZE], int set2[MAX_SIZE], int result[MAX_SIZE]) {
for (int i = 0; i < MAX_SIZE; i++) {
result[i] = set1[i] || set2[i];
}
}
void intersectSet(int set1[MAX_SIZE], int set2[MAX_SIZE], int result[MAX_SIZE]) {
for (int i = 0; i < MAX_SIZE; i++) {
result[i] = set1[i] && set2[i];
}
}
void diffSet(int set1[MAX_SIZE], int set2[MAX_SIZE], int result[MAX_SIZE]) {
for (int i = 0; i < MAX_SIZE; i++) {
result[i] = set1[i] && !set2[i];
}
}
```
运行结果:
```
请输入第一个集合(小写字母):
abc
请输入第二个集合(小写字母):
bcd
第一个集合:{abc}
第二个集合:{bcd}
并集:{abcd}
交集:{bc}
差集(第一个集合-第二个集合):{a}
差集(第二个集合-第一个集合):{d}
```
阅读全文
相关推荐
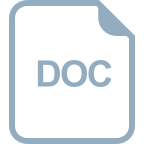
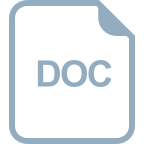




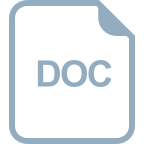

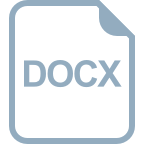
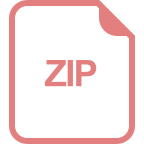
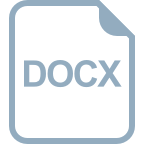
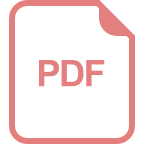
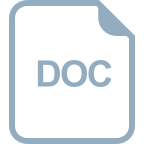
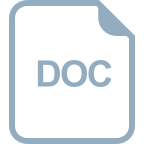
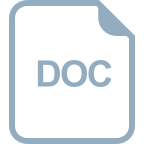
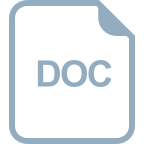
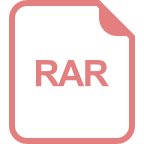
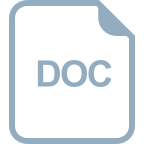
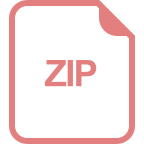