def build_vector(self,text,size,wv): vec = np.zeros(size).reshape(1,size) #创建一个指定大小的数据空间 count = 0#count是统计有多少词向量 for w in text: #循环所有的词向量进行求和 try: vec += wv[w].reshape(1,size) count +=1 except: continue if count!=0:#循环完成后求均值 vec/=count return vecValueError: Expected 2D array, got scalar array instead:array=nan.Reshape your data either using array.reshape(-1, 1) if your data has a single feature or array.reshape(1, -1) if it contains a single sample.
时间: 2024-02-10 12:12:36 浏览: 31
这段代码可能出现了一个错误,错误信息是 "ValueError: Expected 2D array, got scalar array instead: array=nan. Reshape your data either using array.reshape(-1, 1) if your data has a single feature or array.reshape(1, -1) if it contains a single sample." 这个错误通常是因为输入的数据不符合函数的要求,需要使用 reshape 函数将其转换为正确的形状。具体来说,如果输入的数据只有一个特征,那么需要使用 array.reshape(-1, 1) 将其转换为一个二维数组;如果输入的数据只有一个样本,那么需要使用 array.reshape(1, -1) 将其转换为一个二维数组。你可以检查一下输入的数据是否符合函数的要求,如果不符合可以尝试使用 reshape 函数进行转换。
相关问题
解释这段代码# coding: utf-8 from gensim.models.word2vec import Word2Vec import numpy as np import jieba import csv from sklearn.externals import joblib # 对每个句子的所有词向量取均值,来生成一个句子的vector def build_sentence_vector(text, size, imdb_w2v): vec = np.zeros(size).reshape((1, size)) count = 0. for word in text: try: vec += imdb_w2v.wv[word].reshape((1, size)) count += 1. except KeyError: continue if count != 0: vec /= count return vec # 构建待预测句子的向量 def get_predict_vecs(words): n_dim = 300 imdb_w2v = Word2Vec.load(r'..\test\sentiment-analysis\svm_data\w2v_model\w2v_model.pkl') train_vecs = build_sentence_vector(words, n_dim, imdb_w2v) return train_vecs # 对单个句子进行情感判断 def svm_predict(string): words = jieba.lcut(string) words_vecs = get_predict_vecs(words) # 构建测试集的词向量 # 加载训练好的模型 clf = joblib.load(r'..\test\sentiment-analysis\svm_data\svm_model\model.pkl') result = clf.predict(words_vecs) if int(result[0]) == 1: #print("positive") return "1" else: #print("negetive") return "-1" count = 0 prodict = 0 # 计算准确度 with open(r'..\test\sentiment-analysis\test.csv',encoding='utf-8') as csvfile: online = csv.reader(csvfile) for lonly in enumerate(online): count = count + 1 identify = svm_predict(lonly[1][0]) print(lonly[1][1]) if identify == lonly[1][1]: prodict = prodict + 1 accuracy = prodict/count*100.0 print(accuracy)
这段代码的作用是创建一个Python的类,类名为Person,其中包含两个属性name和age,以及两个方法__init__()和get_info()。其中__init__()方法是类的构造函数,用于初始化对象的属性值;get_info()方法用于返回对象的信息,即name和age属性的值。
def avg_feature_vector(sentence, model, num_features, index2word_set): # 定义词向量数量 feature_vec = np.zeros((num_features, ), dtype='float32')#,num_fearures 表示整数或者整数元组,dtype为生成矩阵的数据类型,,numpy.zeros()函数可以满足创建指定长度或形状的全0的数组。 n_words = 0 # 分析句子中每一个词在词库中的情况, for word in str(sentence): word=str(word) if word in index2word_set: n_words += 1 feature_vec = np.add(feature_vec, model.wv[word]) # 进行向量转换 if (n_words > 0): feature_vec = np.divide(feature_vec, n_words) return feature_vec # 将训练集的数据转换为词向量,pandas实现pd,one-hot编码 df=[] for i in range(len(a)): s1_afv = avg_feature_vector(a[i], model=model, num_features=100, index2word_set=index2word_set) df.append(s1_afv) X=pd.DataFrame(df)
这段代码是一个函数定义和一个用于将训练集数据转换为词向量的代码段。
函数名为avg_feature_vector,它接受四个参数:
- sentence:待转换的句子或文本。
- model:用于生成词向量的词嵌入模型。
- num_features:生成的词向量的维度。
- index2word_set:一个集合对象,包含了词库中所有的单词。
该函数首先创建一个全零数组feature_vec,用于保存句子的词向量。然后遍历句子中的每个单词,如果该单词在词库中存在,则将其词向量加到feature_vec中。最后,将feature_vec除以单词数目n_words,得到句子的平均词向量。
接下来的代码是将训练集数据转换为词向量的代码段。首先创建一个空列表df,用于存储转换后的词向量。然后循环遍历训练集中的每个文本,调用avg_feature_vector函数将其转换为词向量,并将结果添加到df列表中。最后,使用pandas库将df列表转换为一个DataFrame对象X,其中每一行代表一个文本的词向量。
相关推荐
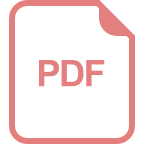
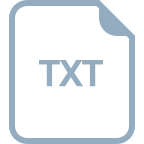













