用c语言基本函数,对文件结构进行分析读入一幅图片像素数据并显示
时间: 2023-05-21 15:03:05 浏览: 156
您好,以下是用C语言基本函数对文件结构进行分析读入一幅图片像素数据并显示的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#pragma pack(1) // 设置结构体按1字节对齐
// BMP文件头结构体
typedef struct {
char type[2]; // 文件类型,必须为"BM"
int size; // 文件大小,单位为字节
short reserved1; // 保留字段,必须为0
short reserved2; // 保留字段,必须为0
int offset; // 数据偏移量,单位为字节
} BMPHeader;
// BMP信息头结构体
typedef struct {
int size; // 信息头大小,单位为字节
int width; // 图像宽度,单位为像素
int height; // 图像高度,单位为像素
short planes; // 颜色平面数,必须为1
short bitsPerPixel; // 每个像素的位数,一般为24
int compression; // 压缩方式,一般为0
int imageSize; // 图像数据大小,单位为字节
int xPixelsPerMeter; // 水平分辨率,单位为像素/米
int yPixelsPerMeter; // 垂直分辨率,单位为像素/米
int colorsUsed; // 使用的颜色数,一般为0
int importantColors; // 重要的颜色数,一般为0
} BMPInfoHeader;
// 读取BMP文件头
void readBMPHeader(FILE* fp, BMPHeader* header) {
fread(header, sizeof(BMPHeader), 1, fp);
}
// 读取BMP信息头
void readBMPInfoHeader(FILE* fp, BMPInfoHeader* infoHeader) {
fread(infoHeader, sizeof(BMPInfoHeader), 1, fp);
}
// 读取BMP像素数据
void readBMPPixelData(FILE* fp, unsigned char* pixelData, int imageSize) {
fread(pixelData, imageSize, 1, fp);
}
// 显示BMP图像
void showBMPImage(unsigned char* pixelData, int width, int height) {
int i, j;
for (i = 0; i < height; i++) {
for (j = 0; j < width; j++) {
printf("(%d, %d, %d) ", pixelData[i * width * 3 + j * 3 + 2], pixelData[i * width * 3 + j * 3 + 1], pixelData[i * width * 3 + j * 3]);
}
printf("\n");
}
}
int main() {
FILE* fp;
BMPHeader header;
BMPInfoHeader infoHeader;
unsigned char* pixelData;
// 打开BMP文件
fp = fopen("test.bmp", "rb");
if (fp == NULL) {
printf("Failed to open file!\n");
return -1;
}
// 读取BMP文件头
readBMPHeader(fp, &header);
// 判断文件类型是否为BM
if (header.type[0] != 'B' || header.type[1] != 'M') {
printf("Invalid file type!\n");
return -1;
}
// 读取BMP信息头
readBMPInfoHeader(fp, &infoHeader);
// 分配像素数据内存
pixelData = (unsigned char*)malloc(infoHeader.imageSize);
if (pixelData == NULL) {
printf("Failed to allocate memory!\n");
return -1;
}
// 读取BMP像素数据
readBMPPixelData(fp, pixelData, infoHeader.imageSize);
// 关闭BMP文件
fclose(fp);
// 显示BMP图像
showBMPImage(pixelData, infoHeader.width, infoHeader.height);
// 释放像素数据内存
free(pixelData);
return 0;
}
```
希望能对您有所帮助!
阅读全文
相关推荐
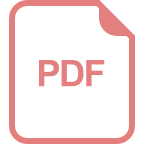
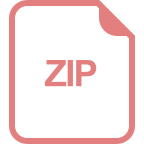











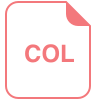
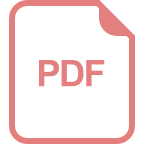
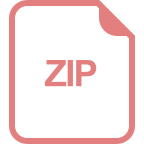