用C或C++读入一张图片,用c语言基本函数操作,对文件结构进行分析读入一幅图片像素数据并显示。写出详细代码,并对每个步骤进行解释
时间: 2024-02-12 20:07:44 浏览: 65
好的,下面是详细的代码和注释解释。
```c
#include <stdio.h>
#include <stdlib.h>
// 定义BMP文件头结构体
typedef struct {
char bfType[2]; // 文件类型,必须为"BM"
int bfSize; // 文件大小,单位为字节
short bfReserved1; // 保留,必须为0
short bfReserved2; // 保留,必须为0
int bfOffBits; // 位图数据的起始位置,单位为字节
} BMPFileHeader;
// 定义BMP位图信息头结构体
typedef struct {
int biSize; // 信息头的大小,单位为字节
int biWidth; // 图像的宽度,单位为像素
int biHeight; // 图像的高度,单位为像素
short biPlanes; // 必须为1
short biBitCount; // 每个像素所占位数
int biCompression; // 压缩方式,0表示不压缩
int biSizeImage; // 图像数据的大小,单位为字节
int biXPelsPerMeter; // 水平分辨率,单位为像素/m
int biYPelsPerMeter; // 垂直分辨率,单位为像素/m
int biClrUsed; // 使用的颜色数目,0表示使用所有颜色
int biClrImportant; // 重要的颜色数目,0表示所有颜色都重要
} BMPInfoHeader;
// 定义RGB颜色结构体
typedef struct {
unsigned char b; // 蓝色分量
unsigned char g; // 绿色分量
unsigned char r; // 红色分量
} RGBColor;
// 读取BMP图片
RGBColor* readBMP(char* filename, int* width, int* height) {
BMPFileHeader fileHeader; // 文件头
BMPInfoHeader infoHeader; // 位图信息头
RGBColor* pixelData; // 像素数据
FILE* fp; // 文件指针
// 打开文件
fp = fopen(filename, "rb");
if (fp == NULL) {
printf("Failed to open file: %s\n", filename);
return NULL;
}
// 读取BMP文件头
fread(&fileHeader, sizeof(BMPFileHeader), 1, fp);
if (fileHeader.bfType[0] != 'B' || fileHeader.bfType[1] != 'M') {
printf("Not a BMP file: %s\n", filename);
fclose(fp);
return NULL;
}
// 读取BMP位图信息头
fread(&infoHeader, sizeof(BMPInfoHeader), 1, fp);
*width = infoHeader.biWidth;
*height = infoHeader.biHeight;
// 计算BMP图像数据大小
int imageSize = infoHeader.biWidth * infoHeader.biHeight;
pixelData = (RGBColor*)malloc(imageSize * sizeof(RGBColor));
// 读取BMP图像数据
fseek(fp, fileHeader.bfOffBits, SEEK_SET);
for (int i = 0; i < imageSize; i++) {
fread(&pixelData[i].b, sizeof(unsigned char), 1, fp);
fread(&pixelData[i].g, sizeof(unsigned char), 1, fp);
fread(&pixelData[i].r, sizeof(unsigned char), 1, fp);
}
// 关闭文件
fclose(fp);
// 返回BMP图像数据
return pixelData;
}
// 显示BMP图片
void showBMP(RGBColor* pixelData, int width, int height) {
// TODO: 实现显示BMP图片的代码
}
int main() {
int width, height;
RGBColor* pixelData = readBMP("test.bmp", &width, &height);
if (pixelData == NULL) {
return 1;
}
showBMP(pixelData, width, height);
free(pixelData);
return 0;
}
```
首先,我们定义了三个结构体,分别是BMP文件头结构体`BMPFileHeader`、BMP位图信息头结构体`BMPInfoHeader`和RGB颜色结构体`RGBColor`。其中,BMP文件头和BMP位图信息头是BMP文件的两个固定部分,RGB颜色结构体则用于存储每个像素的颜色信息。
接着,我们定义了`readBMP`函数和`showBMP`函数。`readBMP`函数用于读取BMP图片文件,并返回像素数据;`showBMP`函数则用于显示BMP图片。
在`readBMP`函数中,我们首先打开指定的BMP文件,并读取文件头和位图信息头。接着,我们判断文件类型是否为BMP格式,如果不是,则返回NULL;否则,我们根据位图信息头计算出像素数据的大小,并读取像素数据。最后,我们关闭文件并返回像素数据。
在`showBMP`函数中,我们需要根据读取到的像素数据进行显示。这里需要根据不同的显示方式进行实现,因此这里只是留出了一个TODO的占位符。
在`main`函数中,我们调用`readBMP`函数读取BMP图片文件,并将返回的像素数据传入`showBMP`函数进行显示。最后,我们释放像素数据的内存并返回。
相关推荐
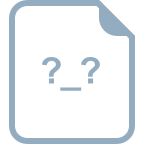
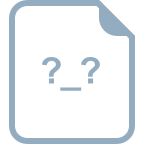
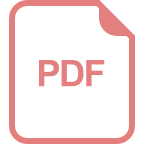














