用c++写个读入obj文件,并输出顶点坐标的程序
时间: 2023-08-31 17:37:21 浏览: 136
### 回答1:
这是一个使用C语言编写的读取obj文件并输出顶点坐标的程序:#include <stdio.h>
#include <stdlib.h>int main(int argc, char *argv[])
{
FILE *fp;
char line[256];
float x, y, z; if(argc < 2)
{
printf("Usage: %s <obj file>\n", argv[0]);
return 1;
} fp = fopen(argv[1], "r");
if(!fp)
{
printf("Error: Could not open file %s\n", argv[1]);
return 1;
} while(fgets(line, 256, fp))
{
if(line[0] == 'v')
{
sscanf(line, "v %f %f %f", &x, &y, &z);
printf("顶点:(%.2f, %.2f, %.2f)\n", x, y, z);
}
} fclose(fp); return 0;
}
### 回答2:
下面是一个用C语言编写的读入obj文件并输出顶点坐标的程序:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_LINE_LENGTH 128
typedef struct {
float x;
float y;
float z;
} Vertex;
int main() {
FILE* file;
char line[MAX_LINE_LENGTH];
Vertex vertex;
// 打开obj文件
file = fopen("example.obj", "r");
if (file == NULL) {
printf("无法打开文件!\n");
return -1;
}
// 读取文件中的每一行
while (fgets(line, MAX_LINE_LENGTH, file)) {
// 如果是顶点坐标行,则打印出顶点坐标
if (line[0] == 'v' && line[1] == ' ') {
sscanf(line, "v %f %f %f", &vertex.x, &vertex.y, &vertex.z);
printf("顶点坐标:(%.2f, %.2f, %.2f)\n", vertex.x, vertex.y, vertex.z);
}
}
// 关闭文件
fclose(file);
return 0;
}
```
该程序使用了一个结构体`Vertex`来存储顶点坐标,每次读取到顶点行时,通过`sscanf`函数将顶点坐标解析到结构体中,并使用`printf`函数输出到控制台上。
请注意,在执行该程序之前需要将要读取的obj文件命名为`example.obj`并与源代码放在同一目录下。
希望这个程序能满足你的需求!如果有任何问题,请随时提问。
### 回答3:
下面是一个使用C语言编写的读取.obj文件并输出顶点坐标的示例程序:
```c
#include <stdio.h>
#include <stdlib.h>
int main() {
FILE *file;
char line[128];
float x, y, z;
// 打开.obj文件
file = fopen("example.obj", "r");
if (file == NULL) {
printf("无法打开文件!\n");
return 0;
}
// 逐行读取文件内容,并输出顶点坐标
while (fgets(line, 128, file) != NULL) {
// 读取顶点数据(以"v "开头的行)
if (strncmp(line, "v ", 2) == 0) {
sscanf(line, "v %f %f %f", &x, &y, &z);
printf("顶点坐标:%.2f %.2f %.2f\n", x, y, z);
}
}
// 关闭文件
fclose(file);
return 0;
}
```
这个程序首先打开名为"example.obj"的.obj文件,然后逐行读取文件内容。如果读取的行以"v "开头,就将该行解析为顶点坐标,并输出这些坐标。最后关闭文件。请将需要读取的.obj文件放在同一个目录下,并将文件名修改为正确的文件名,然后运行该程序即可输出顶点坐标。
阅读全文
相关推荐
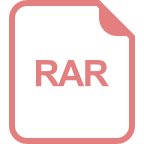















