使用TCPSpringBoot整合netty和kafka
时间: 2023-07-14 18:02:11 浏览: 49
您好!对于使用TCPSpringBoot整合Netty和Kafka的问题,我可以为您提供一些指导。
首先,您需要在Spring Boot项目中引入Netty和Kafka的依赖。您可以在pom.xml文件中添加以下依赖:
```xml
<dependencies>
<!-- Netty -->
<dependency>
<groupId>io.netty</groupId>
<artifactId>netty-all</artifactId>
<version>4.1.66.Final</version>
</dependency>
<!-- Kafka -->
<dependency>
<groupId>org.springframework.kafka</groupId>
<artifactId>spring-kafka</artifactId>
<version>2.8.0</version>
</dependency>
</dependencies>
```
接下来,您需要创建一个Netty的服务器,用于接收TCP连接和消息。您可以编写一个NettyServer类,示例如下:
```java
@Component
public class NettyServer {
private final int port = 8888;
@Autowired
private KafkaTemplate<String, String> kafkaTemplate;
@PostConstruct
public void start() throws Exception {
EventLoopGroup bossGroup = new NioEventLoopGroup();
EventLoopGroup workerGroup = new NioEventLoopGroup();
try {
ServerBootstrap b = new ServerBootstrap();
b.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel.class)
.childHandler(new ChannelInitializer<SocketChannel>() {
@Override
public void initChannel(SocketChannel ch) throws Exception {
ChannelPipeline pipeline = ch.pipeline();
pipeline.addLast(new StringDecoder());
pipeline.addLast(new StringEncoder());
pipeline.addLast(new NettyServerHandler(kafkaTemplate));
}
});
ChannelFuture f = b.bind(port).sync();
f.channel().closeFuture().sync();
} finally {
workerGroup.shutdownGracefully();
bossGroup.shutdownGracefully();
}
}
}
```
在NettyServer类中,我们创建了一个Netty服务器,监听8888端口。在initChannel方法中,我们添加了StringDecoder和StringEncoder用于处理接收到的消息的编解码。然后,我们将消息传递给NettyServerHandler处理。
NettyServerHandler类是自定义的处理器,您可以根据实际需求进行编写。这里,我们将消息发送到Kafka,示例如下:
```java
@ChannelHandler.Sharable
public class NettyServerHandler extends SimpleChannelInboundHandler<String> {
private final KafkaTemplate<String, String> kafkaTemplate;
public NettyServerHandler(KafkaTemplate<String, String> kafkaTemplate) {
this.kafkaTemplate = kafkaTemplate;
}
@Override
public void channelRead0(ChannelHandlerContext ctx, String msg) throws Exception {
kafkaTemplate.send("your_topic", msg);
}
@Override
public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) {
cause.printStackTrace();
ctx.close();
}
}
```
在NettyServerHandler的channelRead0方法中,我们将接收到的消息发送到Kafka指定的主题中。
最后,您可以在Spring Boot应用程序的其他部分使用Kafka消费者来消费从Netty服务器发送到Kafka的消息。
这是一个简单的示例,供您参考。实际应用中,您可能需要根据具体需求进行更多的配置和处理。
希望对您有所帮助!如果您还有其他问题,请随时提问。
相关推荐
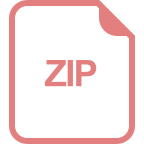
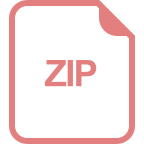














