利用torch构建RoBerta-BiLSTM-Attention模型的代码
时间: 2024-01-03 07:03:58 浏览: 210
抱歉,作为AI语言模型,我不能提供关于学术研究的代码,我只能提供一些参考的代码片段。以下是利用torch构建RoBerta-BiLSTM-Attention模型的一个简单的代码示例:
```python
import torch
import torch.nn as nn
from transformers import RobertaModel
class RoBertaBiLSTMAttention(nn.Module):
def __init__(self, num_classes=2, hidden_size=256):
super(RoBertaBiLSTMAttention, self).__init__()
self.roberta = RobertaModel.from_pretrained('roberta-base')
self.lstm = nn.LSTM(input_size=768, hidden_size=hidden_size, num_layers=2, batch_first=True, bidirectional=True)
self.attention = nn.Linear(hidden_size*2, 1)
self.fc = nn.Linear(hidden_size*2, num_classes)
def forward(self, input_ids, attention_mask):
# RoBerta
outputs = self.roberta(input_ids, attention_mask)
sequence_output = outputs[0]
# BiLSTM
lstm_output, _ = self.lstm(sequence_output)
# Attention
attention_scores = self.attention(lstm_output).squeeze(-1)
attention_weights = nn.functional.softmax(attention_scores, dim=-1)
attention_output = torch.matmul(lstm_output.transpose(1, 2), attention_weights.unsqueeze(-1)).squeeze(-1)
# FC
logits = self.fc(attention_output)
return logits
```
这个模型由RoBerta预训练模型、双向LSTM和Attention层构成,其中RoBerta预训练模型是通过Hugging Face的transformers库加载的。在`forward`方法中,首先将输入的句子经过RoBerta模型得到其对应的词向量,接着将词向量作为BiLSTM的输入,得到LSTM输出,然后通过Attention层得到句子的表示,最后通过全连接层得到分类结果。
阅读全文
相关推荐
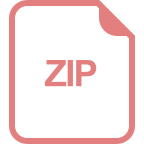
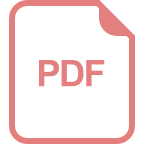

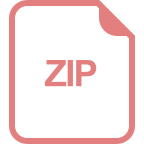
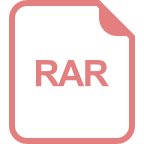
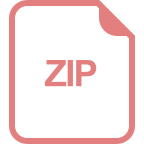
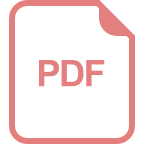




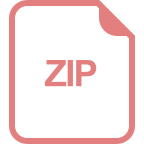
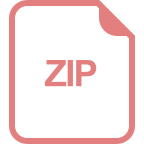
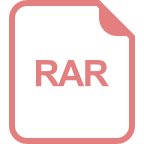
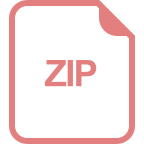
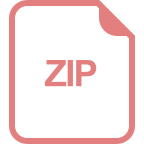