matlab中dbn使用 predict
时间: 2023-11-02 11:04:37 浏览: 36
在 MATLAB 中使用 DBN 进行预测,需要先训练 DBN,然后使用 predict 函数进行预测。以下是一个简单的示例:
```matlab
% 导入数据
load fisheriris
% 将数据分为训练集和测试集
idx = randperm(size(meas,1));
Xtrain = meas(idx(1:100),:);
Ytrain = species(idx(1:100));
Xtest = meas(idx(101:end),:);
Ytest = species(idx(101:end));
% 训练 DBN
numhid = [10 10];
dbn = trainDBN(Xtrain, numhid);
% 预测测试集
Ypred = predict(dbn, Xtest);
% 计算准确率
accuracy = sum(Ypred == Ytest) / numel(Ytest);
```
在上面的示例中,我们首先将数据分为训练集和测试集,然后使用 trainDBN 函数训练 DBN。接着,我们使用 predict 函数对测试集进行预测,并计算预测的准确率。注意,在训练 DBN 时,我们指定了隐藏层的数量和大小。这些参数可以根据实际需求进行调整。
相关问题
matlab中dbn多个参数预测
在Matlab中,DBN(深度信念网络)是一种用于无监督和有监督学习的计算模型。当需要使用DBN进行多个参数的预测时,可以按照以下步骤进行:
1. 数据准备:首先,需要准备好用于训练和测试的多个参数的数据集。确保数据集中的每个参数都有相应的标签或目标值,以便进行有监督的预测。
2. 数据预处理:对于每个参数,可以根据需要进行数据预处理操作,如归一化、标准化或特征选择等。这有助于提高DBN模型的训练和预测性能。
3. DBN模型的构建:在Matlab中,可以使用DeepLearnToolbox等工具箱来构建DBN模型。DBN模型由多个深度信念网络层组成,每个层都由多个神经元组成。可以设置模型的网络结构、层和神经元的数量等超参数。
4. 模型训练:使用准备好的数据集,将数据传入DBN模型进行训练。可以通过设置训练参数(如学习率、迭代次数等)来控制训练过程。训练过程中,DBN会自动学习参数之间的关联和模式。
5. 参数预测:在完成模型训练后,可以使用DBN模型进行多个参数的预测。将测试数据传入DBN模型,模型会根据之前的学习过程预测出参数的值。预测结果可以用于进一步的分析、决策或应用。
需要注意的是,DBN模型的预测准确性受多个因素影响,如模型的训练数据质量、模型结构和参数设置等。因此,在使用DBN进行多个参数预测时,需要进行合适的数据处理和模型调优,以提高预测效果。
matlab中dbn如何设置隐藏层
在Matlab中使用深度信念网络(DBN)时,可以使用以下代码设置隐藏层的数量和大小:
```matlab
% 设置隐藏层的数量和大小
hiddenSizes = [100 50];
% 创建DBN对象
dbn = trainDBN(data, hiddenSizes);
```
其中,`hiddenSizes`是一个包含隐藏层大小的向量,例如`[100 50]`表示有两个隐藏层,第一个隐藏层有100个节点,第二个隐藏层有50个节点。`trainDBN`函数用于训练DBN模型,并返回一个DBN对象。
相关推荐
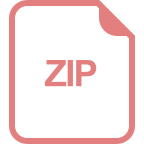
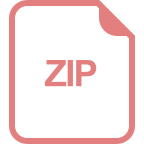












