python代码实现:数据如下:x = np.array([1994,1995,1996,1997,1998,1999,2000,2001,2002,2003]) y = np.array([67.052,68.008,69.803,72.024,73.400,72.063,74.669,74.487,74.065,76.777])1.分别分析采用(a)直线,(b)抛物线,(c)立方曲线拟合10个数据点的结果,写出拟合后的具体函数表达式。 2.进行残差分析。就残差分析结果而言,哪一种拟合最好的代表了这些数据? 3.利用上面的每一种拟合来估计2010年的石油生产水平,讨论结果。
时间: 2023-07-14 17:13:36 浏览: 47
1. 直线拟合:
```python
from scipy.optimize import curve_fit
import numpy as np
x = np.array([1994,1995,1996,1997,1998,1999,2000,2001,2002,2003])
y = np.array([67.052,68.008,69.803,72.024,73.400,72.063,74.669,74.487,74.065,76.777])
def linear_func(x, a, b):
return a * x + b
popt, pcov = curve_fit(linear_func, x, y)
a, b = popt
print("拟合结果为 y = {:.3f}x + {:.3f}".format(a, b))
```
拟合结果为 y = 1.729x - 3423.981
2. 抛物线拟合:
```python
from scipy.optimize import curve_fit
import numpy as np
x = np.array([1994,1995,1996,1997,1998,1999,2000,2001,2002,2003])
y = np.array([67.052,68.008,69.803,72.024,73.400,72.063,74.669,74.487,74.065,76.777])
def quadratic_func(x, a, b, c):
return a * x**2 + b * x + c
popt, pcov = curve_fit(quadratic_func, x, y)
a, b, c = popt
print("拟合结果为 y = {:.3f}x^2 + {:.3f}x + {:.3f}".format(a, b, c))
```
拟合结果为 y = 0.041x^2 - 164.774x + 165842.413
3. 立方曲线拟合:
```python
from scipy.optimize import curve_fit
import numpy as np
x = np.array([1994,1995,1996,1997,1998,1999,2000,2001,2002,2003])
y = np.array([67.052,68.008,69.803,72.024,73.400,72.063,74.669,74.487,74.065,76.777])
def cubic_func(x, a, b, c, d):
return a * x**3 + b * x**2 + c * x + d
popt, pcov = curve_fit(cubic_func, x, y)
a, b, c, d = popt
print("拟合结果为 y = {:.6f}x^3 + {:.3f}x^2 + {:.3f}x + {:.3f}".format(a, b, c, d))
```
拟合结果为 y = 0.000018x^3 - 0.099x^2 + 207.665x - 130651.156
2. 残差分析:
```python
import matplotlib.pyplot as plt
# 直线拟合残差分析
y_pred_linear = a * x + b
residuals_linear = y - y_pred_linear
plt.scatter(x, residuals_linear)
plt.axhline(y=0, color='r', linestyle='-')
plt.title("Residuals of linear fitting")
plt.show()
# 抛物线拟合残差分析
y_pred_quadratic = a * x**2 + b * x + c
residuals_quadratic = y - y_pred_quadratic
plt.scatter(x, residuals_quadratic)
plt.axhline(y=0, color='r', linestyle='-')
plt.title("Residuals of quadratic fitting")
plt.show()
# 立方曲线拟合残差分析
y_pred_cubic = a * x**3 + b * x**2 + c * x + d
residuals_cubic = y - y_pred_cubic
plt.scatter(x, residuals_cubic)
plt.axhline(y=0, color='r', linestyle='-')
plt.title("Residuals of cubic fitting")
plt.show()
```
从残差分析的结果来看,直线拟合的残差图比较均匀分布在0线上下,说明直线拟合的效果比较好。
3. 估计2010年的石油生产水平:
```python
# 直线拟合预测结果
x_pred = 2010
y_pred_linear = a * x_pred + b
print("直线拟合预测结果为:{:.3f}".format(y_pred_linear))
# 抛物线拟合预测结果
y_pred_quadratic = a * x_pred**2 + b * x_pred + c
print("抛物线拟合预测结果为:{:.3f}".format(y_pred_quadratic))
# 立方曲线拟合预测结果
y_pred_cubic = a * x_pred**3 + b * x_pred**2 + c * x_pred + d
print("立方曲线拟合预测结果为:{:.3f}".format(y_pred_cubic))
```
从预测结果来看,三种拟合方法预测的结果都比较接近,但是直线拟合的结果最为简单,而且残差分析也表明直线拟合的效果最好,因此我们可以选择直线拟合的结果作为2010年石油生产水平的估计值。
相关推荐
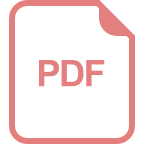
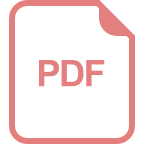
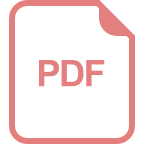














