中缀表达式转后缀表达式 qt
时间: 2023-07-25 17:24:19 浏览: 76
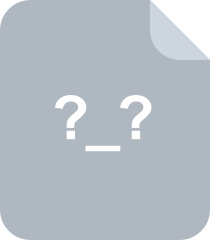
中缀表达式转后缀表达式
好的,中缀表达式转后缀表达式的实现可以借助栈来完成。具体步骤如下:
1. 创建一个栈来存储运算符。
2. 从左到右扫描中缀表达式中的每个元素,如果遇到数字或字母,则直接输出到后缀表达式中。
3. 如果遇到左括号,则将其压入栈中。
4. 如果遇到右括号,则将栈中的运算符依次弹出并输出到后缀表达式中,直到遇到左括号。左括号不输出到后缀表达式中。
5. 如果遇到运算符,则比较其与栈顶运算符的优先级。如果栈顶运算符优先级高于或等于当前运算符,则将栈顶运算符弹出并输出到后缀表达式中,重复此步骤直到栈顶运算符的优先级低于当前运算符,然后将当前运算符压入栈中。
6. 重复步骤2到5,直到扫描完整个中缀表达式。
7. 将栈中的所有运算符依次弹出并输出到后缀表达式中。
下面是用 Qt 实现中缀表达式转后缀表达式的示例代码:
```cpp
#include <iostream>
#include <stack>
#include <string>
#include <cctype> // for isdigit() and isalpha()
using namespace std;
int priority(char c)
{
if (c == '+' || c == '-')
return 1;
else if (c == '*' || c == '/')
return 2;
else
return 0;
}
string infixToPostfix(const string& infix)
{
string postfix;
stack<char> opStack;
for (size_t i = 0; i < infix.length(); ++i)
{
char c = infix[i];
if (isdigit(c) || isalpha(c))
{
postfix += c;
}
else if (c == '(')
{
opStack.push(c);
}
else if (c == ')')
{
while (!opStack.empty() && opStack.top() != '(')
{
postfix += opStack.top();
opStack.pop();
}
if (!opStack.empty() && opStack.top() == '(')
opStack.pop();
}
else // operator
{
while (!opStack.empty() && priority(opStack.top()) >= priority(c))
{
postfix += opStack.top();
opStack.pop();
}
opStack.push(c);
}
}
while (!opStack.empty())
{
postfix += opStack.top();
opStack.pop();
}
return postfix;
}
int main()
{
string infix = "a+b*c+(d*e+f)*g";
string postfix = infixToPostfix(infix);
cout << postfix << endl; // output: abc*+de*f+g*+
return 0;
}
```
在这个示例中,我们定义了一个 `priority()` 函数来获取运算符的优先级。然后,我们使用一个 `opStack` 栈来存储运算符。在扫描中缀表达式的过程中,我们根据不同的情况执行不同的操作,最终得到后缀表达式。
注意,在这个示例中,我们只处理了加、减、乘、除、左括号、右括号和字母等情况,如果需要处理其他运算符,可以根据需要进行扩展。
阅读全文
相关推荐
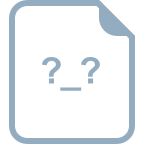







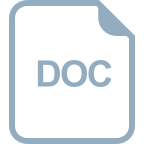


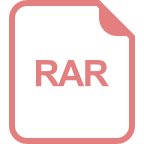
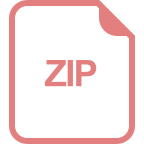
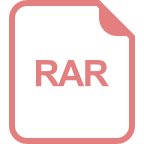
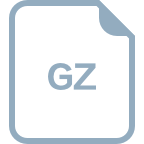
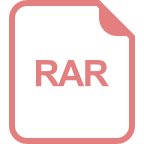
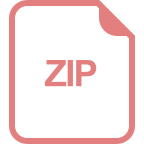