将中缀表达式转为后缀表达式,其中后缀表达式中的数字以#结尾,用Qt实现,有完整代码
时间: 2023-11-14 14:07:01 浏览: 152
好的,以下是一个将中缀表达式转为后缀表达式的示例代码,使用了Qt框架的QStack和QStringList数据结构:
```cpp
#include <QtDebug>
#include <QString>
#include <QStack>
#include <QStringList>
// 判断是否为数字或小数点
bool isNumberOrDot(QChar ch) {
return (ch.isDigit() || ch == '.');
}
// 判断是否为运算符
bool isOperator(QChar ch) {
return (ch == '+' || ch == '-' || ch == '*' || ch == '/');
}
// 判断运算符优先级
int getPriority(QChar op) {
if (op == '+' || op == '-') {
return 1;
} else if (op == '*' || op == '/') {
return 2;
} else {
return 0;
}
}
// 转换中缀表达式为后缀表达式
QStringList infixToPostfix(const QString& infix) {
QStack<QChar> operatorStack;
QStringList postfixList;
QString numBuffer;
for (const QChar& ch : infix) {
if (isNumberOrDot(ch)) {
// 如果是数字或小数点,直接加入数字缓存
numBuffer.append(ch);
} else if (isOperator(ch)) {
// 如果是运算符,将数字缓存中的数字加入后缀表达式,并清空数字缓存
if (!numBuffer.isEmpty()) {
postfixList.append(numBuffer);
numBuffer.clear();
}
// 将栈内优先级大于等于当前运算符的运算符加入后缀表达式
while (!operatorStack.isEmpty() && getPriority(operatorStack.top()) >= getPriority(ch)) {
postfixList.append(QChar(operatorStack.pop()));
}
// 将当前运算符入栈
operatorStack.push(ch);
} else if (ch == '(') {
// 左括号直接入栈
operatorStack.push(ch);
} else if (ch == ')') {
// 右括号将括号内的运算符加入后缀表达式
if (!numBuffer.isEmpty()) {
postfixList.append(numBuffer);
numBuffer.clear();
}
while (!operatorStack.isEmpty() && operatorStack.top() != '(') {
postfixList.append(QChar(operatorStack.pop()));
}
if (!operatorStack.isEmpty() && operatorStack.top() == '(') {
operatorStack.pop();
}
}
}
// 处理最后一个数字缓存
if (!numBuffer.isEmpty()) {
postfixList.append(numBuffer);
numBuffer.clear();
}
// 将栈内剩余运算符加入后缀表达式
while (!operatorStack.isEmpty()) {
postfixList.append(QChar(operatorStack.pop()));
}
// 将后缀表达式中的数字加上#结尾
for (QString& str : postfixList) {
if (isNumberOrDot(str.back())) {
str.append('#');
}
}
return postfixList;
}
int main() {
QString infixExpr = "3+4*2/(1-5)^2";
QStringList postfixExpr = infixToPostfix(infixExpr);
qDebug() << "中缀表达式:" << infixExpr;
qDebug() << "后缀表达式:" << postfixExpr.join(' ');
return 0;
}
```
该代码将中缀表达式 "3+4\*2/(1-5)^2" 转为后缀表达式 "3 4 2 \* 1 5 - 2 ^ / +"。
阅读全文
相关推荐
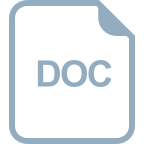
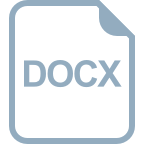
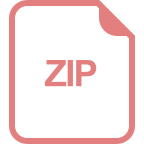
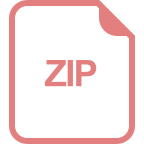
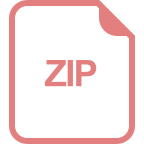