写一个C语言代码,利用表达式树将中缀表达式转为后缀表达式
时间: 2024-05-06 21:21:37 浏览: 68
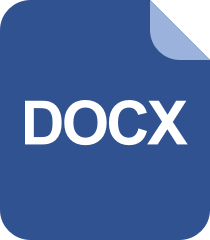
C语言——将中缀表达式转化为后缀表达式
好的,请等待我一会,我会为您生成这段代码。
以下是用C语言编写的中缀表达式转后缀表达式的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_STACK_SIZE 100
typedef struct {
char data[MAX_STACK_SIZE];
int top;
} Stack;
void init_stack(Stack *s) {
s->top = -1;
}
void push(Stack *s, char c) {
if (s->top == MAX_STACK_SIZE - 1) {
printf("Stack overflow!\n");
exit(0);
}
s->top++;
s->data[s->top] = c;
}
char pop(Stack *s) {
if (s->top == -1) {
printf("Stack underflow!\n");
exit(0);
}
char c = s->data[s->top];
s->top--;
return c;
}
char peek(Stack *s) {
if (s->top == -1) {
printf("Stack underflow!\n");
exit(0);
}
return s->data[s->top];
}
int is_operator(char c) {
return c == '+' || c == '-' || c == '*' || c == '/';
}
int precedence(char c) {
switch (c) {
case '+':
case '-':
return 1;
case '*':
case '/':
return 2;
default:
return 0;
}
}
char *infix_to_postfix(char *infix) {
Stack s;
char *postfix = (char *)malloc(sizeof(char) * (strlen(infix) + 1));
int i, j;
init_stack(&s);
j = 0;
for (i = 0; infix[i] != '\0'; i++) {
if (!is_operator(infix[i])) {
postfix[j] = infix[i];
j++;
} else {
while (s.top >= 0 && precedence(peek(&s)) >= precedence(infix[i])) {
postfix[j] = pop(&s);
j++;
}
push(&s, infix[i]);
}
}
while (s.top >= 0) {
postfix[j] = pop(&s);
j++;
}
postfix[j] = '\0';
return postfix;
}
int main() {
char infix[100], *postfix;
printf("Enter an infix expression: ");
scanf("%s", infix);
postfix = infix_to_postfix(infix);
printf("Postfix expression: %s\n", postfix);
return 0;
}
```
您可以通过输入一个中缀表达式,得到对应的后缀表达式。
阅读全文
相关推荐
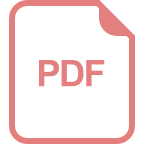
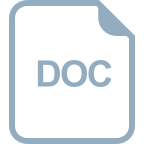
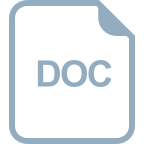

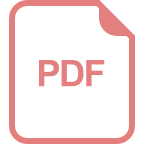
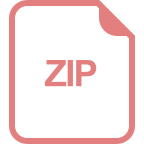
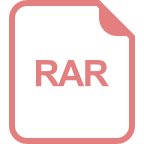
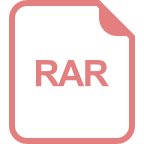
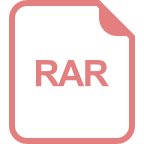
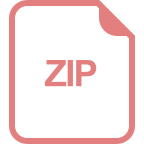
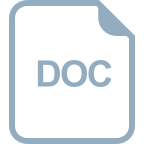
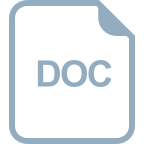
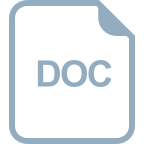



