openSSL实现AES解密
时间: 2024-06-08 20:08:58 浏览: 139
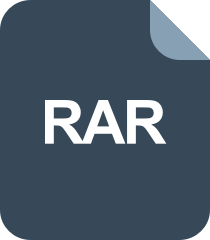
C 语言实现aes加解密
下面是一个使用OpenSSL库实现AES解密的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <openssl/aes.h>
int aes_decrypt(unsigned char *ciphertext, int ciphertext_len, unsigned char *key,
unsigned char *iv, unsigned char *plaintext) {
AES_KEY dec_key;
int len;
if (AES_set_decrypt_key(key, 128, &dec_key) < 0)
return -1;
AES_cbc_encrypt(ciphertext, plaintext, ciphertext_len, &dec_key, iv, AES_DECRYPT);
return 0;
}
int main() {
unsigned char key[AES_BLOCK_SIZE] = "0123456789abcdef"; // 16 bytes key
unsigned char iv[AES_BLOCK_SIZE] = "fedcba9876543210"; // 16 bytes iv
unsigned char ciphertext[] = {0x26, 0xc1, 0x6f, 0x1a, 0x3f, 0x82, 0x20, 0xdd, 0x4c, 0x9b, 0xc0, 0x95, 0x07, 0xec, 0x8a, 0x0e}; // 16 bytes ciphertext
int ciphertext_len = sizeof(ciphertext);
unsigned char plaintext[ciphertext_len];
// Decrypt the ciphertext
aes_decrypt(ciphertext, ciphertext_len, key, iv, plaintext);
// Print the decrypted plaintext
printf("Decrypted plaintext: %s\n", plaintext);
return 0;
}
```
在上述代码中,`aes_decrypt` 函数接受加密后的密文、密钥和初始化向量作为输入,并将解密后的明文输出到 `plaintext` 缓冲区。`main` 函数使用示例密文、密钥和初始化向量调用该函数,并打印出解密后的明文。
请注意,此示例代码中使用的是AES-128算法,因此密钥和初始化向量均为16个字节。如果您使用其他密钥长度,则需要相应地更改其长度。
阅读全文
相关推荐
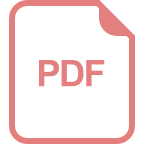
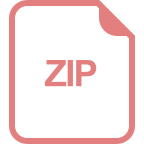















