windows,C语言,编写一段程序,使其用管道来实现父子进程之间的进程通信。子进程向父进程发送自己的进程标识符,以及字符串“is sending a message to parent!”。父进程则通过管道读出子进程发来的消息,将消息显示在屏幕上,然后终止。
时间: 2024-02-12 13:05:59 浏览: 126
好的,以下是你需要的程序代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/wait.h>
#define BUFFER_SIZE 25
#define READ_END 0
#define WRITE_END 1
int main(void)
{
pid_t pid;
int fd[2];
char write_msg[BUFFER_SIZE] = "is sending a message to parent!";
char read_msg[BUFFER_SIZE];
if (pipe(fd) == -1) {
fprintf(stderr,"Pipe failed");
return 1;
}
pid = fork();
if (pid < 0) {
fprintf(stderr, "Fork failed");
return 1;
}
if (pid > 0) {
close(fd[WRITE_END]);
read(fd[READ_END], read_msg, BUFFER_SIZE);
printf("Received message from child: %s\n", read_msg);
close(fd[READ_END]);
} else {
close(fd[READ_END]);
sprintf(write_msg, "%d %s", getpid(), write_msg);
write(fd[WRITE_END], write_msg, strlen(write_msg));
close(fd[WRITE_END]);
}
return 0;
}
```
程序的主要思路是创建一个管道,然后使用 fork() 创建一个子进程。父进程使用管道读取子进程发送的消息,子进程将自己的 PID 和消息写入管道。
在父进程中,首先关闭管道的写端,然后使用 read() 从管道中读取数据,并将其存储在 read_msg 缓冲区中。最后,父进程将读取到的消息打印到屏幕上,然后关闭管道的读端。
在子进程中,首先关闭管道的读端,然后使用 sprintf() 将子进程的 PID 和消息写入 write_msg 缓冲区中。最后,子进程使用 write() 将 write_msg 缓冲区中的内容写入管道,并关闭管道的写端。
这样,子进程就将自己的 PID 和消息写入了管道,父进程就可以读取到这些信息并将消息打印到屏幕上了。
阅读全文
相关推荐
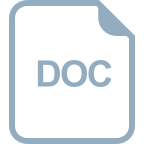
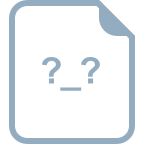






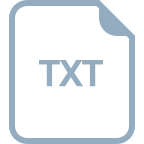
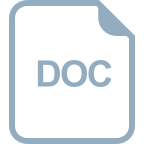
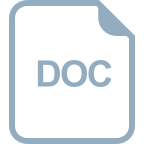
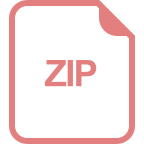
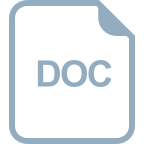
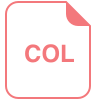
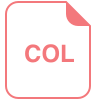
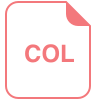
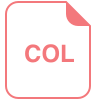