给我一个python程序,可以读写一个jsonlist的文件
时间: 2023-08-31 10:18:44 浏览: 132
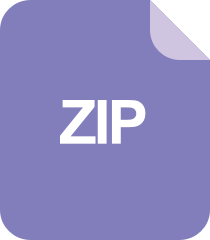
python读取json文件

### 回答1:
当然,我可以回答这个问题。以下是一个示例Python程序,用于读写一个JSON列表文件:
```python
import json
# 读取JSON列表文件
with open('example.json', 'r') as f:
data = json.load(f)
# 打印读取的数据
print(data)
# 向JSON列表文件中写入数据
new_data = [{'name': 'Alice', 'age': 25}, {'name': 'Bob', 'age': 30}]
with open('example.json', 'w') as f:
json.dump(new_data, f)
```
在这个示例程序中,我们使用Python内置的`json`模块来读取和写入JSON格式的数据。`json.load()`函数用于从文件中读取JSON数据,`json.dump()`函数用于将Python数据结构写入JSON文件。
### 回答2:
当然可以!以下是一个可以读写JSON列表文件的Python程序示例:
```python
import json
def read_jsonlist(filename):
with open(filename, 'r') as file:
data = json.load(file)
return data
def write_jsonlist(data, filename):
with open(filename, 'w') as file:
json.dump(data, file)
filename = "example.json"
# 示例数据
data = [{"name": "John", "age": 28}, {"name": "Alice", "age": 32}, {"name": "Bob", "age": 24}]
# 写入数据到JSON文件
write_jsonlist(data, filename)
# 从JSON文件中读取数据
loaded_data = read_jsonlist(filename)
print(loaded_data)
```
在上面的示例中,我们定义了`read_jsonlist(filename)`函数来读取JSON列表文件,并使用`json.load()`函数加载文件内容。然后,我们定义了`write_jsonlist(data, filename)`函数来将数据写入JSON文件,并使用`json.dump()`函数将数据转换成JSON格式并写入文件。最后,我们使用示例数据调用这两个函数,并打印读取的结果。
你可以将上述代码保存为一个Python文件,例如`jsonlist.py`,然后运行它以测试读写功能。确保你已经安装了Python和`json`模块。
希望这个程序能够满足你的需求!如有任何进一步的问题,请随时提问。
### 回答3:
以下是一个可以读写一个jsonlist文件的Python程序:
```python
import json
# 读取jsonlist文件
def read_jsonlist(filename):
try:
with open(filename, 'r') as file:
data = json.load(file)
return data
except FileNotFoundError:
print("文件不存在")
return []
# 写入jsonlist文件
def write_jsonlist(filename, data):
with open(filename, 'w') as file:
json.dump(data, file)
# 示例用法
my_list = [{'name': 'Alice', 'age': 25}, {'name': 'Bob', 'age': 30}]
# 写入jsonlist文件
write_jsonlist('data.json', my_list)
# 读取jsonlist文件
new_list = read_jsonlist('data.json')
# 输出读取结果
print(new_list)
```
以上程序定义了两个函数`read_jsonlist`和`write_jsonlist`,分别用于读取和写入jsonlist文件。使用`json`模块的`load`函数读取jsonlist文件,使用`json`模块的`dump`函数写入jsonlist文件。
程序示例创建了一个名为`my_list`的列表,其中包含两个字典元素。首先使用`write_jsonlist`函数将`my_list`写入名为`data.json`的文件中,然后使用`read_jsonlist`函数读取该文件,最后打印读取的结果。
你可以根据实际需要修改`my_list`的内容和文件名,并在程序中适应不同的操作。
阅读全文
相关推荐
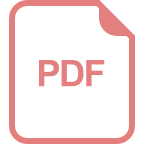
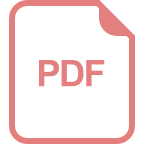
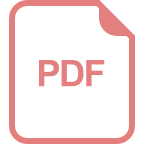
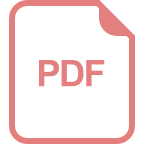
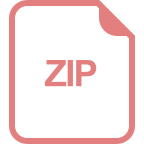
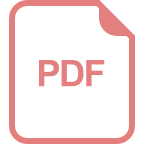
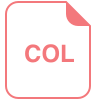
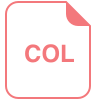









