c语言【问题描述】 请编制函数实现功能:在字符串(其长度小于80)中统计“字符串中英文字母的个数”,函数返回“英文字母的个数”;若无字母,则返回0;并用相应主函数进行测试。通过命令行参数接收“被处理的字符串”。 注意:如果命令行接收参数个数多于或小于2,则可以直接判定为输入错误,输出“Input error,end.”(输出内容不含双引号)。 【输入形式】 输入“被处理的字符串”。 输入是从命令行输入。 【输出形式】 输出被处理字符串的英文字母的个数;输
时间: 2024-01-22 22:17:35 浏览: 77
下面是 C 语言的实现代码:
```
#include <stdio.h>
#include <string.h>
int count_letters(char *str) {
int count = 0;
for (int i = 0; i < strlen(str); i++) {
if ((str[i] >= 'a' && str[i] <= 'z') || (str[i] >= 'A' && str[i] <= 'Z')) {
count++;
}
}
return count;
}
int main(int argc, char *argv[]) {
if (argc != 2) {
printf("Input error,end.\n");
return 0;
}
int count = count_letters(argv[1]);
printf("%d\n", count);
return 0;
}
```
该程序首先检查命令行参数的数量是否为2,如果不是,则输出错误信息并退出。如果是,则调用 `count_letters` 函数计算字符串中的英文字母数量,并输出结果。
函数 `count_letters` 遍历字符串中每个字符,如果是英文字母则计数器加1。最后返回计数器的值。
相关问题
【问题描述】 请编制函数实现功能:在字符串(其长度小于80)中统计“字符串中英文字母的个数”,函数返回“英文字母的个数”;若无字母,则返回0;并用相应主函数进行测试。通过命令行参数接收“被处理的字符串”。 注意:如果命令行接收参数个数多于或小于2,则可以直接判定为输入错误,输出“Input error,end.”(输出内容不含双引号)。 【输入形式】 输入“被处理的字符串”。 输入是从命令行输入。 【输出形式】 输出被处理字符串的英文字母的个数;输入错误时,输出“Input error,end.”(输出内容不含双引号)。 【样例输入1】 123abc!@#cdf 【样例输出1】 The string:123abc!@#cdf. The number of English letters:6. 【样例输入2】 abc 123 【样例输出2】 Input error,end. 【样例输入3】 123 【样例输出3】 The string:123. The number of English letters:0.这个题用C语言怎么做?
可以使用以下程序实现:
```c
#include <stdio.h>
#include <ctype.h>
#include <string.h>
int count_letters(char *s) {
int count = 0;
for (int i = 0; i < strlen(s); i++) {
if (isalpha(s[i]))
count++;
}
return count;
}
int main(int argc, char *argv[]) {
if (argc != 2) {
printf("Input error,end.\n");
} else {
char *s = argv[1];
int num_letters = count_letters(s);
printf("The string:%s.\tThe number of English letters:%d.\n", s, num_letters);
}
return 0;
}
```
其中,`count_letters` 函数用来统计一个字符串中英文字母的个数;主程序中,首先检查命令行参数的个数是否为 2,如果不是,则输出错误信息;否则,将第一个命令行参数作为字符串进行处理,并输出结果。需要注意的是,在 C 语言中,字符串是以字符数组的形式存储的,所以需要使用 `strlen` 函数来获取字符串的长度。
C语言函数fun的功能是:统计字符串中英文字母、空格、数字和其它字符的个数
以下是C语言函数fun的实现,可以统计字符串中英文字母、空格、数字和其它字符的个数:
```c
#include <stdio.h>
#include <ctype.h>
void fun(char *str, int *a, int *b, int *c, int *d) {
while (*str != '\0') {
if (isalpha(*str)) {
(*a)++;
} else if (*str == ' ') {
(*b)++;
} else if (isdigit(*str)) {
(*c)++;
} else {
(*d)++;
}
str++;
}
}
int main() {
char str[100];
int a = 0, b = 0, c = 0, d = 0;
printf("请输入一个字符串:");
gets(str);
fun(str, &a, &b, &c, &d);
printf("英文字母个数:%d\n", a);
printf("空格个数:%d\n", b);
printf("数字个数:%d\n", c);
printf("其它字符个数:%d\n", d);
return 0;
}
```
阅读全文
相关推荐
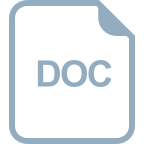
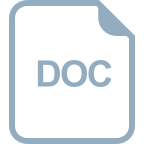
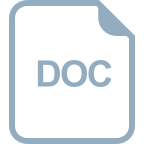













