autograd.function嵌套nn.module
时间: 2024-06-08 08:07:32 浏览: 193
在PyTorch中,`nn.Module`是一个用于神经网络构建的基类。`autograd.Function`则是一个用于定义自定义操作的基类。这两个类在PyTorch中都有重要的作用。
当我们需要定义一个自定义操作时,可以使用`autograd.Function`类来实现。在实现自定义操作时,我们需要定义一个`forward`方法和一个`backward`方法。`forward`方法用于计算前向传播,`backward`方法则用于计算反向传播。在`backward`方法中,我们可以使用PyTorch提供的自动微分机制来计算梯度。
当我们需要定义一个神经网络结构时,可以使用`nn.Module`类来实现。在实现神经网络结构时,我们需要定义一个`forward`方法。在`forward`方法中,我们可以使用PyTorch提供的各种已有操作来构建神经网络。同时,我们也可以使用自定义操作来扩展PyTorch的功能。
在实际使用中,我们有时候需要在`nn.Module`中使用自定义操作。这时,可以将自定义操作嵌套在`nn.Module`中。具体来说,我们可以在`nn.Module`的构造函数中定义自定义操作,并在`forward`方法中使用该自定义操作。这样,我们就可以在神经网络中使用自定义操作了。
例如,下面是一个简单的示例,演示了如何在`nn.Module`中嵌套`autograd.Function`:
```python
import torch
from torch.autograd import Function
from torch import nn
class CustomFunction(Function):
@staticmethod
def forward(ctx, input):
# 计算前向传播
output = input * 2
# 保存中间结果,以备反向传播使用
ctx.save_for_backward(input)
return output
@staticmethod
def backward(ctx, grad_output):
# 获取保存的中间结果
input, = ctx.saved_tensors
# 计算反向传播
grad_input = grad_output * 2
return grad_input
class CustomModule(nn.Module):
def __init__(self):
super(CustomModule, self).__init__()
self.custom_function = CustomFunction()
def forward(self, x):
# 在forward中使用自定义操作
y = self.custom_function(x)
return y
# 测试代码
x = torch.tensor([1.0, 2.0, 3.0], requires_grad=True)
module = CustomModule()
y = module(x)
z = y.sum()
z.backward()
print(x.grad)
```
在上面的示例中,我们定义了一个名为`CustomFunction`的自定义操作,并将其嵌套在了`CustomModule`中。在`CustomModule`的`forward`方法中,我们使用了`CustomFunction`来计算前向传播。在`CustomFunction`的`backward`方法中,我们使用了PyTorch提供的自动微分机制来计算梯度。
最后,我们对`z`进行反向传播,得到了`x`的梯度。可以看到,`x`的梯度为`torch.tensor([2.0, 2.0, 2.0])`,与我们的预期一致。
阅读全文
相关推荐
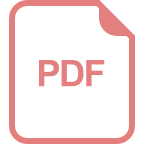
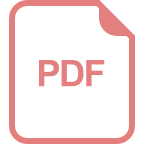
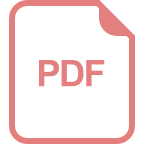











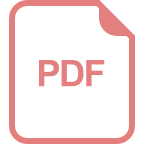



