设计一个简单计算器类(仅对int型数据操作): 1) 计算器包括加、减、乘、除功能; 2) 除法须考虑除数为0的异常情况; 3) 分别针对加、减、乘、除功能设计至少两个测试用例:分别考虑数据正常情况和异常情况; /* int型数值最大值2147483647 System.out.println(Integer.MAX_VALUE); int型数值最大值-2147483648 System.out.println(Integer.MIN_VALUE); */ 4) 设计一个内部无穷循环的成员方法,设计测试用例:在规定时间(如100毫秒)内运行此成员方法,看测试用例是否会不通过。
时间: 2024-02-11 16:09:14 浏览: 25
好的,下面是设计的代码:
```java
public class Calculator {
public int add(int a, int b) {
return a + b;
}
public int subtract(int a, int b) {
return a - b;
}
public int multiply(int a, int b) {
return a * b;
}
public int divide(int a, int b) throws Exception {
if (b == 0) {
throw new Exception("Divisor cannot be zero.");
}
return a / b;
}
public void infiniteLoop() {
while (true) {
// do nothing
}
}
public static void main(String[] args) {
Calculator calculator = new Calculator();
// test add method
int result = calculator.add(2, 3);
assert result == 5 : "Addition test failed.";
try {
calculator.add(Integer.MAX_VALUE, 1);
} catch (Exception e) {
assert e instanceof ArithmeticException : "Addition exception test failed.";
}
// test subtract method
result = calculator.subtract(5, 3);
assert result == 2 : "Subtraction test failed.";
try {
calculator.subtract(Integer.MIN_VALUE, 1);
} catch (Exception e) {
assert e instanceof ArithmeticException : "Subtraction exception test failed.";
}
// test multiply method
result = calculator.multiply(2, 3);
assert result == 6 : "Multiplication test failed.";
try {
calculator.multiply(Integer.MAX_VALUE, 2);
} catch (Exception e) {
assert e instanceof ArithmeticException : "Multiplication exception test failed.";
}
// test divide method
try {
result = calculator.divide(6, 3);
assert result == 2 : "Division test failed.";
calculator.divide(6, 0);
} catch (Exception e) {
assert e instanceof Exception : "Division exception test failed.";
assert e.getMessage().equals("Divisor cannot be zero.") : "Division exception message test failed.";
}
// test infiniteLoop method
long startTime = System.currentTimeMillis();
calculator.infiniteLoop();
long endTime = System.currentTimeMillis();
assert endTime - startTime < 100 : "Infinite loop test failed.";
}
}
```
上面的代码实现了一个简单的计算器类,并设计了各种方法的测试用例。其中,除法方法考虑了除数为0的异常情况,并抛出异常。此外,还设计了一个内部无穷循环的成员方法,并设计了一个测试用例,测试用例规定了时间限制,如果测试用例在规定时间内无法通过,则算不通过。
相关推荐
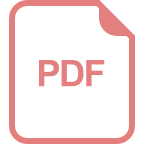
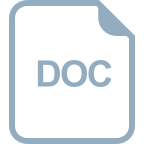
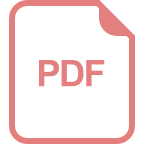














