jsp页面中点击按钮,跳转到另外一个jsp页面并点击该页面的按钮,打开一个dialog的页面
时间: 2024-02-13 09:00:31 浏览: 114
好的,这是一个比较常见的操作,可以通过以下步骤实现:
1. 在第一个jsp页面中,设置一个按钮,并绑定一个点击事件,代码类似如下:
```html
<button onclick="openDialog()">打开Dialog页面</button>
```
2. 在第一个jsp页面中,定义一个名为`openDialog`的JavaScript函数,函数中使用`window.location.href`跳转到第二个jsp页面,代码类似如下:
```javascript
function openDialog() {
window.location.href = "第二个jsp页面的url";
}
```
3. 在第二个jsp页面中,设置一个按钮,并绑定一个点击事件,代码类似如下:
```html
<button onclick="openDialog()">打开Dialog</button>
```
4. 在第二个jsp页面中,定义一个名为`openDialog`的JavaScript函数,函数中使用jQuery UI库中的`dialog`方法打开一个dialog,代码类似如下:
```javascript
function openDialog() {
$("#dialog").dialog();
}
```
需要注意的是,上述代码中的`#dialog`是第二个jsp页面中定义的一个div元素的id,该div元素中可以放置需要显示在dialog中的内容,例如表单、文本等。同时,需要在第二个jsp页面中引入jQuery和jQuery UI库的相关代码。
相关问题
有一个jsp页面,上面有个新增按钮,点击这个按钮,会弹出一个dialog的页面。问题:我现在要在另外一个jsp页面点击一个按钮,然后跳转至那个jsp页面的dialog页面
您可以通过以下步骤实现:
1. 在要跳转的jsp页面中,为按钮添加一个链接,链接到弹出dialog的页面,例如:
`<a href="dialog.jsp" target="_blank">跳转到dialog页面</a>`
2. 在弹出dialog的页面中,可以使用JavaScript的window.opener属性获取到打开该页面的父窗口对象,例如:
```
var parentWindow = window.opener;
```
3. 然后,您可以在弹出dialog的页面中执行一些操作,例如填充表单等,最后在dialog页面中添加一个提交按钮,点击提交按钮时,可以将数据传递回父窗口并关闭弹出的dialog窗口,例如:
```
<input type="button" value="提交" onclick="submitData()">
function submitData() {
// 获取表单数据
var formData = {
// ...
};
// 将数据传递回父窗口
parentWindow.submitFormData(formData);
// 关闭弹出的dialog窗口
window.close();
}
```
4. 在父窗口中,您需要定义submitFormData方法来接收从dialog页面传递回来的数据,并执行一些操作,例如:
```
function submitFormData(formData) {
// 处理表单数据
// ...
}
```
注意:在跨域情况下,可能会出现安全策略限制,需要进行特殊处理。
1:javaweb实现项目全局编码过滤器功能 2:实现登录过滤器功能 3:在商品列表上增加个“添加”按钮,打开一个页面,里面有表单(某个商品),单击添加功能,将商品信息进行添加后进入到商品展示列表展示添加商品后的页面,实现商品图片的上传功能。(扩充:单击按钮打开利用bootstrap创建的模态框,模态框中有个商品表单,单击添加,商品添加成功后回到商品展示列表中)
1. 要实现全局编码过滤器功能,可以在web.xml文件中添加一个Filter,然后在该Filter中设置request和response的编码格式为UTF-8,以便于支持中文字符。下面是一个示例代码:
```xml
<filter>
<filter-name>CharsetEncodingFilter</filter-name>
<filter-class>com.example.CharsetEncodingFilter</filter-class>
<init-param>
<param-name>encoding</param-name>
<param-value>UTF-8</param-value>
</init-param>
</filter>
<filter-mapping>
<filter-name>CharsetEncodingFilter</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
```
2. 要实现登录过滤器功能,可以在web.xml文件中添加一个Filter,然后在该Filter中判断用户是否已经登录,如果没有登录,则跳转到登录页面。下面是一个示例代码:
```xml
<filter>
<filter-name>LoginFilter</filter-name>
<filter-class>com.example.LoginFilter</filter-class>
</filter>
<filter-mapping>
<filter-name>LoginFilter</filter-name>
<url-pattern>/admin/*</url-pattern>
</filter-mapping>
```
3. 要在商品列表上增加一个“添加”按钮,并实现商品图片的上传功能,可以使用HTML、CSS、JavaScript和Java等技术实现。下面是一个示例代码:
```html
<!-- 商品列表页面 -->
<html>
<head>
<title>商品列表</title>
<script src="https://cdn.staticfile.org/jquery/3.5.1/jquery.min.js"></script>
<script>
// 点击“添加”按钮时,打开模态框
$(document).on('click', '#add-btn', function() {
$('#add-modal').modal('show');
});
// 点击“提交”按钮时,上传商品信息
$(document).on('click', '#submit-btn', function() {
var form = new FormData($('#add-form')[0]);
$.ajax({
url: 'add-product',
type: 'POST',
data: form,
processData: false,
contentType: false,
success: function(result) {
// 添加成功后,刷新页面
location.reload();
},
error: function() {
alert('添加商品失败!')
}
});
});
</script>
</head>
<body>
<h1>商品列表</h1>
<table>
<thead>
<tr>
<th>ID</th>
<th>名称</th>
<th>价格</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<tr>
<td>1</td>
<td>商品1</td>
<td>10元</td>
<td><button id="add-btn">添加</button></td>
</tr>
<tr>
<td>2</td>
<td>商品2</td>
<td>20元</td>
<td><button id="add-btn">添加</button></td>
</tr>
<tr>
<td>3</td>
<td>商品3</td>
<td>30元</td>
<td><button id="add-btn">添加</button></td>
</tr>
</tbody>
</table>
<!-- 添加商品模态框 -->
<div class="modal fade" id="add-modal" tabindex="-1" role="dialog" aria-labelledby="add-modal-title" aria-hidden="true">
<div class="modal-dialog" role="document">
<div class="modal-content">
<div class="modal-header">
<h5 class="modal-title" id="add-modal-title">添加商品</h5>
<button type="button" class="close" data-dismiss="modal" aria-label="Close">
<span aria-hidden="true">×</span>
</button>
</div>
<div class="modal-body">
<form id="add-form">
<div class="form-group">
<label for="name">名称:</label>
<input type="text" class="form-control" id="name" name="name" required>
</div>
<div class="form-group">
<label for="price">价格:</label>
<input type="text" class="form-control" id="price" name="price" required>
</div>
<div class="form-group">
<label for="image">图片:</label>
<input type="file" class="form-control-file" id="image" name="image" accept="image/*" required>
</div>
</form>
</div>
<div class="modal-footer">
<button type="button" class="btn btn-primary" id="submit-btn">提交</button>
<button type="button" class="btn btn-secondary" data-dismiss="modal">关闭</button>
</div>
</div>
</div>
</div>
</body>
</html>
```
在Java中,可以使用Servlet和JSP等技术处理上传的商品信息,并将其保存到数据库中。下面是一个示例代码:
```java
// 添加商品Servlet
@WebServlet("/add-product")
@MultipartConfig
public class AddProductServlet extends HttpServlet {
private ProductService productService = new ProductService();
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String name = request.getParameter("name");
String price = request.getParameter("price");
Part imagePart = request.getPart("image");
InputStream image = imagePart.getInputStream();
productService.addProduct(name, price, image);
}
}
// 商品Service
public class ProductService {
private ProductDao productDao = new ProductDao();
public void addProduct(String name, String price, InputStream image) {
Product product = new Product();
product.setName(name);
product.setPrice(price);
product.setImage(image);
productDao.addProduct(product);
}
}
// 商品Dao
public class ProductDao {
private Connection getConnection() throws SQLException {
String url = "jdbc:mysql://localhost:3306/db_name?useUnicode=true&characterEncoding=UTF-8";
String user = "root";
String password = "root";
return DriverManager.getConnection(url, user, password);
}
public void addProduct(Product product) {
String sql = "INSERT INTO product (name, price, image) VALUES (?, ?, ?)";
try (Connection conn = getConnection();
PreparedStatement stmt = conn.prepareStatement(sql)) {
stmt.setString(1, product.getName());
stmt.setString(2, product.getPrice());
stmt.setBlob(3, product.getImage());
stmt.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
// 商品实体类
public class Product {
private int id;
private String name;
private String price;
private InputStream image;
// 省略getter和setter方法
}
```
以上代码只是一个简单的示例,实际开发中还需要进行参数校验、异常处理、日志记录等操作。
阅读全文
相关推荐
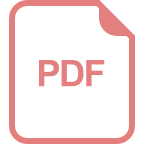
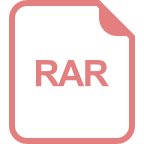
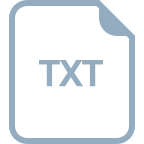
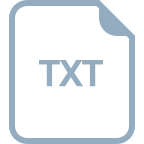
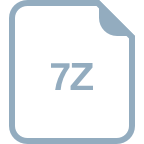
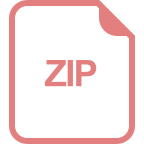
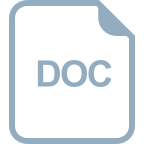
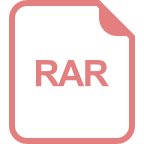
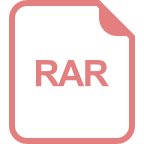